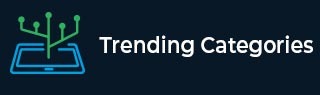
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How will you explain Python for-loop to list comprehension?
List comprehensions offer a concise way to create lists based on existing lists. When using list comprehensions, lists can be built by leveraging any iterable, including strings and tuples. list comprehensions consist of an iterable containing an expression followed by a for the clause. This can be followed by additional for or if clauses.
Let’s look at an example that creates a list based on a string:
hello_letters = [letter for letter in 'hello'] print(hello_letters)
This will give the output:
['h', 'e', 'l', 'l', 'o']
string hello is iterable and the letter is assigned a new value every time this loop iterates. This list comprehension is equivalent to:
hello_letters = [] for letter in 'hello': hello_letters.append(letter)
You can also put conditions on the comprehension. For example,
hello_letters = [letter for letter in 'hello' if letter != 'l'] print(hello_letters)
This will give the output:
['h', 'e', 'o']
You can perform all sorts of operations on the variable. For example,
squares = [i ** 2 for i in range(1, 6)] print(squares)
This will give the output:
[1, 4, 9, 16, 25]
There are a lot more use cases of these comprehensions. They are pretty expressive and useful. You can learn more about them on https://www.digitalocean.com/community/tutorials/understanding-list-comprehensions-in-python-3.