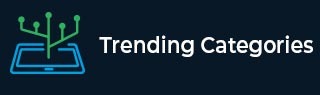
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to work with document.links in JavaScript?
In this tutorial, let us discuss how to work with the document's link in JavaScript.
The document link property is a read-only DOM level 1 feature that returns all the links. The links property gives all the anchor elements and area tags with a href attribute.
Working with document.links properties
Let us learn to work with a link's properties.
Users can follow the syntax below to work with the link's properties.
Syntax
let links = document.links; links.propertyName;
The above syntax returns all anchor tags, area tags, and properties.
Properties
length − The length is the number of elements in an HTML collection.
Return Value
The link property returns the HTML link object collection. The object follows the source code order.
Example
The program below tries to access all the link properties. The code uses a try-catch block to handle errors when we access invalid links properties.
We have four links in this example. But the property returns only two because the remaining links do not have a href attribute.
The anchor link1 and the area link1 are the valid links in this example. We display valid anchor link's the inner HTML, href, and the inner text properties and valid area tag's href property using the [index] method.
<html> <body> <h2> Working with the document link's properties </h2> <a name="docAncLink1"> Anchor Link1 </a> <br> <br> <a href="https://tutorialspoint.com"> Anchor Link2</a> <br> <br> <area name="docAreaLink1" href="https://docAreaLink1.com"> Area Link1 </area> <br> <br> <area id="docAreaLink2"> Area Link2 </area> <br> <br> <div id="docLinkBtnWrap"> <button id="docLinkBtn"> Click Me </button> </div> <p id="docLinkOut"> </p> <script> var docLinkInp = document.getElementById("docLinkInp"); var docLinkOut = document.getElementById("docLinkOut"); var docLinkBtnWrap = document.getElementById("docLinkBtnWrap"); var docLinkBtn = document.getElementById("docLinkBtn"); var docLinkInpStr = ""; docLinkBtn.onclick = function() { docLinkInpStr = ""; let docLinkNode = document.links; //docLinkBtnWrap.style.display = "none"; try { docLinkInpStr += "<br><br><b>The total valid links using length property = </b>" + docLinkNode.length; docLinkInpStr += "<br><br><b>The first valid link innerHTML = </b>" + docLinkNode[0].innerHTML; docLinkInpStr += "<br><br><b>The first valid link href = </b>" + docLinkNode[0].href; docLinkInpStr += "<br><br><b>The first valid link inner text = </b>" + docLinkNode[0].innerText; docLinkInpStr += "<br><br><b>The second valid link href = </b>" + docLinkNode[1].href; } catch (e) { docLinkInpStr += "<br><br>" + e; } docLinkOut.innerHTML = docLinkInpStr; }; </script> </body> </html>
Working with document.links methods
Let us learn to work with a link's methods.
Users can follow the syntax below to work with the link's methods.
Syntax
let links = document.links; links.methodName;
The above syntax returns all anchor tags, area tags, and methods.
Methods
[index] − The index method returns the element at the specific position. The index starts from zero. The method returns "null" if the index is out of range.
item(index) − Returns the element at the specific position. The index starts from zero. The method returns "null" if the index is out of range.
namedItem(id) − Returns the element with the specific id. The method returns "null" if the id is wrong.
Example
The program below tries to access the link's properties using the methods available.
The anchor link and the area link in this program are valid. We use the [index] method to access the anchor link's text. The namedItem("name") method returns the anchor link href property value. The item(index) method returns the anchor link name.
The namedItem("name") and item(index) return the href value of the area link.
<html> <body> <h2> Work with the document link's methods </h2> <a name="linkAnchorMeth" href="https://tutorialspoint.com"> Anchor Link </a> <br> <br> <area name="linkAreaMeth" href="https://totorix.com"> Area Link </a> <br> <br> <div id="linkMethBtnWrap"> <button id="linkMethBtn"> Click Me </button> </div> <p id="linkMethOut"> </p> <script> var linkMethInp = document.getElementById("linkMethInp"); var linkMethOut = document.getElementById("linkMethOut"); var linkMethBtnWrap = document.getElementById("linkMethBtnWrap"); var linkMethBtn = document.getElementById("linkMethBtn"); var linkMethInpStr = ""; linkMethBtn.onclick = function() { linkMethInpStr = ""; let linkMethNode = document.links; //linkMethBtnWrap.style.display = "none"; try { linkMethInpStr += "<br><br><b>The anchor link text using [index] = </b>" + linkMethNode[0].text; linkMethInpStr += "<br><br><b>The anchor link href using namedItem() </b>" + linkMethNode.namedItem("linkAnchorMeth").href; linkMethInpStr += "<br><br><b>The anchor link name using item() </b>" + linkMethNode.item(0).name; linkMethInpStr += "<br><br><b>The area link href using [index] = </b>" + linkMethNode[1].href; linkMethInpStr += "<br><br><b>The area link href using namedItem() </b>" + linkMethNode.namedItem("linkAreaMeth").href; linkMethInpStr += "<br><br><b>The area link href using item() </b>" + linkMethNode.item(1).href; } catch (e) { linkMethInpStr += "<br><br>" + e; } linkMethOut.innerHTML = linkMethInpStr; }; </script> </body> </html>
This tutorial taught us to work with a link's properties and methods. All the properties and methods are built-in by JavaScript.