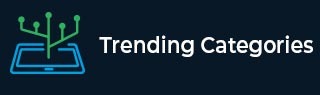
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to validate a given number in JavaScript?
In this tutorial, let us discuss how to validate a given number in JavaScript. Users can follow the syntax below each method to use it.
Using regular expression
The method tests or matches the regular expression with the input.
Syntax
/\D/.test(input); input.match(/^[0-9]*$/);
If the input is a number, the test returns true.
Example
The program succeeds in all cases except for "30", [], and "".
<html> <body> <h2>Number validation using <i>regular expression</i></h2> <p id="numRegExpInp"></p> <div id="numRegExpBtnWrap"> <button id="numRegExpBtn">Validate</button> </div> <p id="numRegExpOut"></p> <script> var numRegExpInpArr = [30, 'Egan', true, false, 'undefined', 'NaN', '{}', null]; var numRegExpInp = document.getElementById("numRegExpInp"); var numRegExpOut = document.getElementById("numRegExpOut"); var numRegExpBtnWrap = document.getElementById("numRegExpBtnWrap"); var numRegExpBtn = document.getElementById("numRegExpBtn"); var numRegExpInpStr = ""; numRegExpInpStr += JSON.stringify(numRegExpInpArr); numRegExpInp.innerHTML = numRegExpInpStr; function numRegExpMatch(input) { if ((/^[0-9]*$/).test(input)) return "correct"; else return "wrong"; } numRegExpBtn.onclick = function() { numRegExpInpStr = ""; //numRegExpBtnWrap.style.display = "none"; numRegExpInpStr += numRegExpInpArr[0] + " is " + numRegExpMatch(numRegExpInpArr[0]) + "<br><br>"; numRegExpInpStr += "'" + numRegExpInpArr[1] + "'" + " is " + numRegExpMatch(numRegExpInpArr[1]) + "<br><br>"; numRegExpInpStr += numRegExpInpArr[2] + " is " + numRegExpMatch(numRegExpInpArr[2]) + "<br><br>"; numRegExpInpStr += numRegExpInpArr[3] + " is " + numRegExpMatch(numRegExpInpArr[3]) + "<br><br>"; numRegExpInpStr += numRegExpInpArr[4] + " is " + numRegExpMatch(undefined) + "<br><br>"; numRegExpInpStr += numRegExpInpArr[5] + " is " + numRegExpMatch(NaN) + "<br><br>"; numRegExpInpStr += numRegExpInpArr[6] + " is " + numRegExpMatch({}) + "<br><br>"; numRegExpInpStr += numRegExpInpArr[7] + " is " + numRegExpMatch(numRegExpInpArr[7]) + "<br><br>"; numRegExpOut.innerHTML = numRegExpInpStr; }; </script> </body> </html>
Using the typeof() operator
The typeof() operator checks the input type.
Syntax
typeof(input) === 'number'
If the type is a number, the check returns true.
Example
The program succeeds in all cases except for NaN.
<html> <body> <h2>Number validation using <i>typeof()</i></h2> <p id="numTypeInp"></p> <div id="numTypeBtnWrap"> <button id="numTypeBtn">Validate</button> </div> <p id="numTypeOut"></p> <script> var numTypeInpArr = [30, '30', 'Egan', true, false, 'undefined', '{}', '[]', null, '']; var numTypeInp = document.getElementById("numTypeInp"); var numTypeOut = document.getElementById("numTypeOut"); var numTypeBtnWrap = document.getElementById("numTypeBtnWrap"); var numTypeBtn = document.getElementById("numTypeBtn"); var numTypeInpStr = ""; numTypeInpStr += JSON.stringify(numTypeInpArr); numTypeInp.innerHTML = numTypeInpStr; function numTypeMatch(input) { if (typeof(input) === "number") return "correct"; else return "wrong"; } numTypeBtn.onclick = function() { numTypeInpStr = ""; //numTypeBtnWrap.style.display = "none"; numTypeInpStr += numTypeInpArr[0] + " is " + numTypeMatch(numTypeInpArr[0]) + "<br><br>"; numTypeInpStr += "'" + numTypeInpArr[1] + "'" + " is " + numTypeMatch(numTypeInpArr[1]) + "<br><br>"; numTypeInpStr += "'" + numTypeInpArr[2] + " is " + numTypeMatch(numTypeInpArr[2]) + "<br><br>"; numTypeInpStr += numTypeInpArr[3] + " is " + numTypeMatch(numTypeInpArr[3]) + "<br><br>"; numTypeInpStr += numTypeInpArr[4] + " is " + numTypeMatch(numTypeInpArr[4]) + "<br><br>"; numTypeInpStr += numTypeInpArr[5] + " is " + numTypeMatch(undefined) + "<br><br>"; numTypeInpStr += numTypeInpArr[6] + " is " + numTypeMatch({}) + "<br><br>"; numTypeInpStr += numTypeInpArr[7] + " is " + numTypeMatch([]) + "<br><br>"; numTypeInpStr += numTypeInpArr[8] + " is " + numTypeMatch(numTypeInpArr[8]) + "<br><br>"; numTypeInpStr += "''" + " is " + numTypeMatch('') + "<br><br>"; numTypeOut.innerHTML = numTypeInpStr; }; </script> </body> </html>
Using the isNaN() object
The isNaN is the browser window's global object.
Syntax
!isNaN(input);
The above syntax checks whether the input is not a number. Negation of this check says whether the input is a number.
Example
The program succeeds in all cases except for "30", true, false, [], null, "";
<html> <body> <h2>Number validation using <i>isNaN()</i></h2> <p id="numNanInp"></p> <div id="numNanBtnWrap"> <button id="numNanBtn">Validate</button> </div> <p id="numNanOut"></p> <script> var numNanInpArr = [30, 'Egan', 'undefined', 'NaN', '{}']; var numNanInp = document.getElementById("numNanInp"); var numNanOut = document.getElementById("numNanOut"); var numNanBtnWrap = document.getElementById("numNanBtnWrap"); var numNanBtn = document.getElementById("numNanBtn"); var numNanInpStr = ""; numNanInpStr += JSON.stringify(numNanInpArr); numNanInp.innerHTML = numNanInpStr; function numNanMatch(input) { if (isNaN(input)) return "wrong"; else return "correct"; } numNanBtn.onclick = function() { numNanInpStr = ""; numNanBtnWrap.style.display = "none"; numNanInpStr += numNanInpArr[0] + " is " + numNanMatch(numNanInpArr[0]) + "<br><br>"; numNanInpStr += "'" + numNanInpArr[1] + "'" + " is " + numNanMatch(numNanInpArr[1]) + "<br><br>"; numNanInpStr += numNanInpArr[2] + " is " + numNanMatch(undefined) + "<br><br>"; numNanInpStr += numNanInpArr[3] + " is " + numNanMatch(NaN) + "<br><br>"; numNanInpStr += numNanInpArr[4] + " is " + numNanMatch({}) + "<br><br>"; numNanOut.innerHTML = numNanInpStr; }; </script> </body> </html>
Using the Number.isFinite() method
The function isFinite() checks whether the input is finite. The method first converts the input to a number and checks whether it is finite.
The method ensures that the input is a number, not a positive or negative infinity, and not NaN.
Syntax
Number.isFinite(input);
The above syntax checks whether the input is finite.
Example
The program succeeds in all cases.
<html> <body> <h2>Number validation using <i>Number.isFinite()</i></h2> <p id="numFiniteInp"></p> <div id="numFiniteBtnWrap"> <button id="numFiniteBtn">Validate</button> </div> <p id="numFiniteOut"></p> <script> var numFiniteInpArr = [30, '30', 'Egan', true, false, 'undefined', 'NaN', '{}', '[]', null, '']; var numFiniteInp = document.getElementById("numFiniteInp"); var numFiniteOut = document.getElementById("numFiniteOut"); var numFiniteBtnWrap = document.getElementById("numFiniteBtnWrap"); var numFiniteBtn = document.getElementById("numFiniteBtn"); var numFiniteInpStr = ""; numFiniteInpStr += JSON.stringify(numFiniteInpArr); numFiniteInp.innerHTML = numFiniteInpStr; function numFiniteMatch(input) { if (Number.isFinite(input)) return "correct"; else return "wrong"; } numFiniteBtn.onclick = function() { numFiniteInpStr = ""; numFiniteBtnWrap.style.display = "none"; numFiniteInpStr += numFiniteInpArr[0] + " is " + numFiniteMatch(numFiniteInpArr[0]) + "<br><br>"; numFiniteInpStr += "'" + numFiniteInpArr[1] + "'" + " is " + numFiniteMatch(numFiniteInpArr[1]) + "<br><br>"; numFiniteInpStr += "'" + numFiniteInpArr[2] + " is " + numFiniteMatch(numFiniteInpArr[2]) + "<br><br>"; numFiniteInpStr += numFiniteInpArr[3] + " is " + numFiniteMatch(numFiniteInpArr[3]) + "<br><br>"; numFiniteInpStr += numFiniteInpArr[4] + " is " + numFiniteMatch(numFiniteInpArr[4]) + "<br><br>"; numFiniteInpStr += numFiniteInpArr[5] + " is " + numFiniteMatch(undefined) + "<br><br>"; numFiniteInpStr += numFiniteInpArr[6] + " is " + numFiniteMatch(NaN) + "<br><br>"; numFiniteInpStr += numFiniteInpArr[7] + " is " + numFiniteMatch({}) + "<br><br>"; numFiniteInpStr += numFiniteInpArr[8] + " is " + numFiniteMatch([]) + "<br><br>"; numFiniteInpStr += numFiniteInpArr[9] + " is " + numFiniteMatch(numFiniteInpArr[9]) + "<br><br>"; numFiniteInpStr += "''" + " is " + numFiniteMatch('') + "<br><br>"; numFiniteOut.innerHTML = numFiniteInpStr; }; </script> </body> </html>
Using the Number() Method
The function Number() checks whether the number is valid.
Syntax
Number(input);
The above syntax checks the number directly.
Example
The program succeeds in all cases except for "30", true, false, [], null, "";
<html> <body> <h2>Number validation using <i>Number()</i></h2> <p id="numMethInp"></p> <div id="numMethBtnWrap"> <button id="numMethBtn">Validate</button> </div> <p id="numMethOut"></p> <script> var numMethInpArr = [30, 'Egan', false, 'undefined', 'NaN', '{}', '[]', null, '']; var numMethInp = document.getElementById("numMethInp"); var numMethOut = document.getElementById("numMethOut"); var numMethBtnWrap = document.getElementById("numMethBtnWrap"); var numMethBtn = document.getElementById("numMethBtn"); var numMethInpStr = ""; numMethInpStr += JSON.stringify(numMethInpArr); numMethInp.innerHTML = numMethInpStr; function numMethMatch(input) { if (Number(input)) return "correct"; else return "wrong"; } numMethBtn.onclick = function() { numMethInpStr = ""; numMethBtnWrap.style.display = "none"; numMethInpStr += numMethInpArr[0] + " is " + numMethMatch(numMethInpArr[0]) + "<br><br>"; numMethInpStr += "'" + numMethInpArr[1] + "'" + " is " + numMethMatch(numMethInpArr[1]) + "<br><br>"; numMethInpStr += numMethInpArr[2] + " is " + numMethMatch(numMethInpArr[2]) + "<br><br>"; numMethInpStr += numMethInpArr[3] + " is " + numMethMatch(undefined) + "<br><br>"; numMethInpStr += numMethInpArr[4] + " is " + numMethMatch(NaN) + "<br><br>"; numMethInpStr += numMethInpArr[5] + " is " + numMethMatch({}) + "<br><br>"; numMethInpStr += numMethInpArr[6] + " is " + numMethMatch([]) + "<br><br>"; numMethInpStr += numMethInpArr[7] + " is " + numMethMatch(numMethInpArr[7]) + "<br><br>"; numMethInpStr += "''" + " is " + numMethMatch('') + "<br><br>"; numMethOut.innerHTML = numMethInpStr; }; </script> </body> </html>
This tutorial taught us five options to validate a number. The Number.isFinite() method is the best among all. Every other method fails in some or more scenarios.