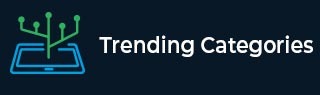
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to understand JavaScript module pattern?
In this article we are going to learn how to understand JavaScript module pattern.
The Module pattern is used to copy the concept of classes, so that we can store both public and private methods and variables inside a single object.
Since JavaScript doesn’t support classes, similar to classes that are used in other programming languages like Java or Python.
Why use modules?
Maintainability − A module is self-contained and aims to lessen the dependencies, so that it can grow and improve independently.
Name spacing − In JavaScript, variables outside the scope of a top-level function are global. Because of this, it’s common to have “namespace pollution”, were completely unrelated code shares global variables.
Reusability − Use the code we previously wrote into new projects at one point or another, some utility methods you wrote from a previous project to your current project.
Encapsulation − The module pattern encapsulates and wraps public & private methods & variables and protects private ways and properties to leak in global scopes. This returns only public methods and public variables.
With module, we can have private functions and variables which can be referenced only within the module and can be referenced other modules. These are advanced objectoriented solutions to commonly occurring software issues.
So, a good understanding of JavaScript patterns, helps us to choose the correct pattern for problem and lets us understand the actual value for your application.
Example
Following is the example program to understand module pattern in JavaScript.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body> <script> (function () { var Age = [10, 20,15, 50, 27, 17, 22, 87, 65, 45]; var average = function() { var total = Age.reduce(function(accumulator, age) { return accumulator + age}, 0); return total / Age.length + '.'; } var notQualified = function(){ var notAdult = Age.filter(function(age) { return age < 18;}); return 'age less than 18 = ' + notAdult.length; } document.write(notQualified()); }()); </script> </body> </html>
We can also change the array list and can retrieve the correct output.
Example
Following is another example program to understand module pattern in JavaScript.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body> <script> var counter=(function(){ let count=0;//private function print(message){ document.write(message+'---'+count, "<br>"); } return{ // value:count, get: function(){return count;}, set:function(value){ count=value;}, increment:function(){ count+=1; print("after increment") }, reset:function(){ print('before increment'); count=0; print('after reset'); } } })(); counter.increment(); counter.increment(); counter.set(7); document.write("Counter Value: ",counter.get(),"<br>"); counter.reset(); </script> </body> </html>