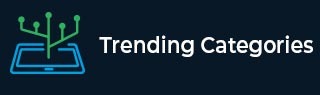
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to split at NEWLINEs in Python?
In this article, we are going to find out how to split at newlines in Python.
In general, split means to divide or to make a group of objects divide into smaller groups.
The first approach to split at NEWLINES in python is by using the inbuilt method splitlines(). It takes a multi-line string as input and it returns a separated string that is divided at the new line. It doesn’t take any parameters.
The splitlines() method is an inbuilt string method and it is used only for splitting at the new lines.
Example 1
In the program given below, we are taking a multi-line string as input and we are splitting the string at newline using the splitlines() method −
str1 = "Welcome\nto\nTutorialspoint" print("The given string is") print(str1) print("The resultant string split at newline is") print(str1.splitlines())
Output
The output of the above example is given below −
The given string is Welcome to Tutorialspoint The resultant string split at newline is ['Welcome', 'to', 'Tutorialspoint']
Example 2
In the example given below, we are using the same splitlines() method for splitting at the newline but we are taking input in a different fashion −
str1 = """Welcome To Tutorialspoint""" print("The given string is") print(str1) print("The resultant string split at newline is") print(str1.splitlines())
Output
The output of the above example is as shown below −
The given string is Welcome to Tutorialspoint The resultant string split at newline is ['Welcome', 'to', 'Tutorialspoint']
Using split() method
The second approach is by using the inbuilt method split(). We need to mention one parameter, the character at which we want the given string to split. So, if we want to split at the new line, we should give ‘\n’ as a parameter. The split() method can be used to split at any character, unlike the splitlines() method. We just need to send the character at which we would like the string to be split.
Example 1
In the example given below, we are taking a string as input and we are dividing the string at a new line using split() method −
str1 = "Welcome\nto\nTutorialspoint" print("The given string is") print(str1) print("The resultant string split at newline is") print(str1.split('\n'))
Output
The output of the above example is given below −
The given string is Welcome to Tutorialspoint The resultant string split at newline is ['Welcome', 'to', 'Tutorialspoint']
Example 2
In the example given below, we are taking the same program as above but we are taking a different substring and checking. −
str1 = """Welcome To Tutorialspoint""" print("The given string is") print(str1) print("The resultant string split at newline is") print(str1.split('\n'))
Output
The output of the above example is given below −
The given string is Welcome to Tutorialspoint The resultant string split at newline is ['Welcome', 'to', 'Tutorialspoint']