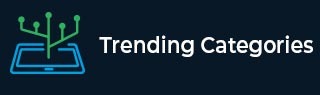
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to simulate a keypress event in JavaScript?
We use event handlers to simulate keypress events in JavaScript. An event in JavaScript, as the name suggests, refers to actions taken by the browser or user. An event handler is a utility that JavaScript provides, that lets the programmer implement the correct course of response to those events.
We will be using the jQuery library to simulate keypress events in JavaScript. It is a library for JavaScript to traverse HTML documents as well as manipulate them, create animations, and much more. One such feature is event handling that we will be using.
Event
An Event is a change that happens in the browser or system. A mouse click is assessed as a change in the system, and so it is noted as an event. Examples of other events are a key pressed on the keyboard, a form loaded, and a web page finishing loading.
There are mainly four types of events each concerned with a different source of input for an event. These are Mouse Events, Keyboard Events, Document Events, and Form Events.
Examples
- Mouse Events - click, dblclick
- Keyboard Events - keypress, keyup
- Document Events - load, resize
- Form Events – submit, change
Now the information of this event is passed on to the programmer by attaching event handlers to the concerned elements of the HTML document.
Syntax
We use selectors in jQuery for attaching event handlers to different HTML tags, classes, and ids. Selectors are part of the jQuery framework that makes it easy to navigate as well as manipulate HTML documents.
$(selector).keypress(function)
Where selector can be a class, id, or tag. This line attaches a keypress handler to the provided selector.
This pattern of referencing various HTML elements in the document using selectors is a common feature of jQuery. We will be using it to refer to other parts of the script but in a different way than JavaScript due to the added abstraction of jQuery.
Example 1
Here we are going to handle a keypress event on the body of an HTML document. Whenever a key is pressed on the browser window, it is logged on the screen.
Before attaching an event handler, we first check whether the DOM (Document Object Model) is ready or not. This is done with the help of jQuery(document).ready() function. When the state of readiness is achieved, the block of code provided as the argument inside ready() runs. Here we will provide a function that attaches the event handler to the body of the HTML document.
Let’s look at the code for the same.
<html> <head> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"> </script> <script> // checks for the readiness of the DOM jQuery(document).ready(function($) { // attaches a keypress handler to body $("body").keypress(function() { document.getElementById("result").innerHTML = "Key Pressed"; }); }); </script> </head> <body> <div id = "result"> press any key </div> </body> </html>
In the above code, we refer to the body of the HTML document using the selector pattern of jQuery and attach a keypress handler to it.
We can even capture the key that has been pressed on the keyboard and log that on the screen.
Log Keystrokes on the Screen
Example 2
In the code below, we first attach a keypress handler to the body element of the HTML document. Then we create a function in jQuery that triggers a keypress event and takes a default character as an argument. The character is ‘Z’ in this case.
We will then use the setTimeout method to call this function after every 3 seconds, which logs the ‘Z’ key on the screen.
One interesting thing to note here is that the keypress handler is attached to the body of the document, so any key press from the user side will get logged on the screen as well.
<html> <head> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"> </script> <script> jQuery(document).ready(function($) { $("body").keypress(function(e) { // logs the pressed key on the screen document.getElementById("result").innerHTML = String.fromCharCode( e.which ) + " key has been pressed."; }); }); // function to trigger the keypress jQuery.fn.simulateKeyPress = function(character) { jQuery(this).trigger({ type: 'keypress', which: character.charCodeAt(0) }); }; // calls the simulateKeypress function every 3 second setTimeout(function() { $("body").simulateKeyPress('Z'); }, 3000); </script> </head> <body> <div id = "result">Wait for the simulation to begin !</div> <p> Press any key </p> </body> </html>
Conclusion
Events in JavaScript make our life easier as a programmer. They provide us with the right tools to handle the various events occurring in the system.