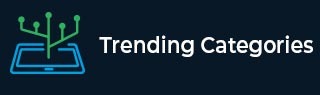
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to set the indentation of the first line of text with JavaScript?
In this tutorial, we will learn how to set the indentation of the first line of text with JavaScript.
The indentation of the first line of a text is a common way to start a new paragraph. To set the indentation, use the textIndent property.
To set the indentation of the first line of text with JavaScript, we will discuss two different ways −
Using the style.textIndent Property
Using the style.setProperty Method
Using the style.textIndent Property
The style.textIndent property of an element is used to set the indentation of the first line of text. This property is placed in the element object, so we use the document.getElementById() method to access it, and then we use the style.textIndent property to set the indentation.
Syntax
document.getElementById('element_id').style.textIndent = 'length | % | inherit | initial'
In the above syntax, the ‘element_id’ is the id attribute of an element. The document.getElementById() method is used to access the element and style.textIndent property is used to set the indentation of the first line of text.
Parameters
length − The length of the indentation in units.
% − The indentation length in percentage(%) of the parent element's width.
inherit − Its parent element’s property inherits the text-indent property.
initial − The text-indent property is set to default.
Example
In the below example, we have used the style.textIndent property to set the indentation of the first line of text in JavaScript. The button “Set Indentation” is associated with a click event that executes the “setTextIndentation()” function that sets the indentation of the first line of text.
<html> <body> <h2> Indenting the first line of text using the <i> style.textIndent property </i> in JavaScript </h2> <div> <button onclick="setTextIndentation()"> Set Indentation </button> <div id="root" style="border: 2px solid gray; background-color: aliceblue; padding: 10px; margin: 5px 0px;"> Welcome to Tutorialspoint. This is a demo paragraph to demonstrate the indentation of the first line of text! </div> <script> // 'Set Indentation' button click event handler function function setTextIndentation() { const root = document.getElementById('root') root.style.textIndent = '50px' } </script> </body> </html>
Using the style.setProperty() Method
In JavaScript, the style.setProperty method sets a property of an element, either new or existing. Firstly, the document.getElementById() method is used to access the element and then set the ‘text-indent’ property using the style.setProperty method. In the property name parameter of the style.setProperty method should be ‘text-indent’, and the value and priority will be as per the user’s requirement.
Syntax
document.getElementById('element_id').style.setProperty(property_name, value, priority)
In the above syntax, the document.getElementById() method is used to access the element object of an HTML element that has the id attribute sets as ‘element_id’, and then we use the style.setProperty method on that element object.
Parameters
property_name − The name of the property to be set.
value − The new value of the property.
priority − The priority of the property value (optional).
Example
In the below example, we have used the style.setProperty method to set the indentation of the first line of text with JavaScript. An input field is taken to get the user’s input for the length of the indentation of the text. The button “Set Indentation” is associated with a click event that executes the “setTextIndentation()” function that sets the indentation of the first line of text as per the user’s input.
<html> <body> <h2> Indenting the first line of text using the <i> style.setProperty method </i> in JavaScript </h2> <div> <h4> Enter the length of the indentation (in px or %): </h4> <input type="text" name="indentation" id="indentation" value="40px"> <button onclick="setTextIndentation()"> Set Indentation </button> <div id="root" style="border: 2px solid gray; background-color: aliceblue; padding: 10px; margin: 5px 0px;"> Welcome to Tutorialspoint. This is a demo paragraph to demonstrate the indentation of the first line of text. The indentation length will be sets by the input field's value! </div> <script> // 'Set Indentation' button click event handler function function setTextIndentation() { const root = document.getElementById('root') // user input value for the length of the indentation const indentation = document.getElementById('indentation').value root.style.setProperty('text-indent', indentation) } </script> </body> </html>
In this tutorial, we learned how to set the indentation of the first line of text with JavaScript. We used two approaches, one with the style.textIndent property and another with the style.setProperty() method. We have also seen two examples where we set a predefined value and a user-input value for the length of the text indentation. Users can use any of these approaches as per their requirements.