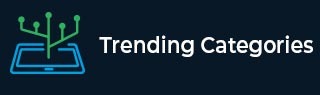
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to set the color of the top border with JavaScript?
In this tutorial, we will learn how to set the color of the top border with JavaScript.
The HTML element’s outline is called the border. The border of an element can have multiple colors on each side. Different properties and methods are used to color each side of the border. To set the color of the top border with JavaScript, we have different ways −
Using the style.borderTopColor Property
Using the style.borderColor Property
Using the style.borderTopColor Property
The style.borderTopColor property of an element in JavaScript is used to specify the color of the element's top border. The style.borderTopColor property is available on the element object that is accessed by the document.getElementById() method. After accessing the element object, we directly use the style.borderTopColor property sets the color of the top border of an element.
Syntax
const object = document.getElementById('id') object.style.borderTopColor = 'color | transparent | inherit | initial'
Here, ‘id’ is the id attribute of an element. The element object is accessed by using the document.getElementById() method and sets the style.borderTopColor property to color the top border.
Parameters
color − The top border color of the element.
transparent − The top border color should be transparent.
inherit − The top border color is inherited from its parent element’s property.
initial − The top border color sets to default.
Example
In the below example, we have used the style.borderTopColor property to set the color of the top border with JavaScript. A button “Set Top Border Color” is associated with a click event that executes a function “setTopBorderColor()” that sets the top border color of multiple elements.
<html> <head> <style> div { border: 5px solid transparent; padding: 10px; margin: 10px 0; background-color: rgb(251, 255, 196); } </style> </head> <body> <p> Set the color of the top border using <i> style.borderTopColor </i> property with JavaScript </p> <button onclick="setTopBorderColor()"> Set Top Border Color </button> <div id="element1"> JavaScript is Best! </div> <div id="element2"> Hello World! </div> <div id="element3"> Welcome to Tutorialspoint! </div> <div id="element4"> It is transparent border color! </div> <script> // all elements that will set the top border color const element1 = document.getElementById('element1') const element2 = document.getElementById('element2') const element3 = document.getElementById('element3') const element4 = document.getElementById('element4') // 'Set Top Border Color' button click event handler function function setTopBorderColor() { element1.style.borderTopColor = 'red' element2.style.borderTopColor = 'green' element3.style.borderTopColor = 'blue' element4.style.borderTopColor = 'transparent' } </script> </body> </html>
Using the style.borderColor Property
The style.borderColor property of an element is used to specify the element's border color in JavaScript. The style.borderColor property is available in the element object of an element. To set the top border color, we need to access the element object, and after that, we can directly set the style.borderColor property. To set only the top border color, we must set all the other sides' border colors transparent.
Syntax
const object = document.getElementById('id') object.style.borderColor = 'color | transparent | inherit | initial'
In the above syntax, ‘id’ is the id attribute of an HTML element. Using the document.getElementById() method we are accessing the element object and set the style.borderColor property.
Parameters
color − The border color of the element.
transparent − The border color should be transparent.
inherit − The border color inherits its parent element’s property.
initial − The border color sets to default.
Example
In the below example, we have used the style.borderColor property to set the color of the top border with JavaScript. We have used an input field to take the user’s input for the border color as a color name, hex color code, or RGB color code and set that color as the element’s border color using a button click event.
<html> <body> <h3> Set the color of the top border using <i> style.borderColor </i> property with JavaScript </h3> <p>Write the top border color to set:</p> <input type="text" name="border-color" id="border-color" value="green"> <button onclick="setTopBorderColor()"> Set Top Border Color </button> <div id="root" style="border: 5px solid transparent; padding: 10px; margin: 10px 0; background-color: rgb(251, 255, 196);"> This is an element! </div> <script> // 'Set Top Border Color' button click event handler function function setTopBorderColor() { const root = document.getElementById('root') // border-color input field value const border_color = document.getElementById('border-color').value // set the border-color input fields value to element's top border color root.style.borderColor = border_color + ' transparent transparent transparent' } </script> </body> </html>
In this tutorial, we learned how to set the color of the top border with JavaScript. We have seen two ways to color the top border using the style.borderTopColor and the style.borderColor properties. Users can follow any of these methods to color the top border of an element.