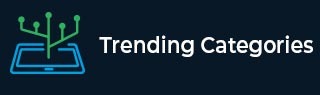
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to set the bottom position of 3D elements with JavaScript?
In this tutorial, we will learn how to set the bottom position of 3D elements with JavaScript. In a web page, handling a 3D element can be tricky. Using JavaScript, we had to set the top, bottom, sides, etc. To set the bottom position of 3D elements, we use the perspectiveOrigin property of an element’s style object.
Using style.perspectiveOrigin Property
In JavaScript, the style.perspectiveOrigin property is used to set the bottom position or base position of a 3D element. Firstly, we get the element object using the document.getElementById() method and then set the style.perspectiveOrigin property.
Syntax
const object = document.getElementById('element_id') object.style.perspectiveOrigin = "x-axis y-axis | inherit | initial"
In the above syntax, ‘element_id’ is the id of a 3D element. We are accessing the element using the document.getElementById() method and then we are setting the bottom using style.perspectiveOrigin property.
Parameters
x-axis − The position in the x-axis of the element. Default value 50%.
y-axis − The position in the y-axis of the element. Default value 50%.
inherit − Inherits its parent element’s property.
initial − Sets to default.
Example 1
You can try to run the following code to set the bottom position of 3D elements with JavaScript. We have three DIVs in the code.
<!DOCTYPE html> <html> <head> <style> #div1 { position: relative; margin: auto; height: 200px; width: 200px; padding: 20px; border: 2px solid blue; perspective: 100px; } #div2 { padding: 80px; position: absolute; border: 2px solid BLUE; background-color: yellow; transform: rotateX(45deg); } #div3 { padding: 50px; position: absolute; border: 2px solid BLUE; background-color: green; transform: rotateX(30deg); } </style> </head> <body> <p>Change the property of div</p> <button onclick = " display ()">Set Perspective Origin Position</button> <div id = "div1">DIV1 <div id = "div2">DIV2</div> <div id = "div3">DIV3</div> </div> <script> function display() { document.getElementById("div1").style.perspectiveOrigin = "20px 10%"; } </script> </body> </html>
Example 2
In the below example, we have used the style.perspectiveOrigin property to set the bottom position of 3D elements with JavaScript by user input. We have used an input field to take the user input to set the bottom position of 3D elements. The “Set Perspective Origin Position” function is executed whenever we click on the “Set Perspective Origin Position” button. The function first gets the input field’s value by the document.getElementById() method and value property and then set the style.perspectiveOrigin property with the input field’s value.
<html> <head> <style> #div1 { position: relative; margin: auto; height: 150px; width: 150px; padding: 20px; border: 2px solid black; perspective: 180px; } #div2 { padding: 80px; position: absolute; border: 2px solid black; background-color: limegreen; transform: rotateX(45deg); } #div3 { padding: 50px; position: absolute; border: 2px solid black; background-color: cornflowerblue; transform: rotateX(30deg); } </style> </head> <body> <p>Fill the x-axis and y-axis positions (with %) below and click the "Set Perspective Origin Position" button to set the bottom position of the 3D element</p> <input id="input_field" type="text" name="bottom" placeholder = "10% 60%"><br> <button onclick = "setBottom()"> Set Perspective Origin Position </button> <div id = "div1"> DIV1 <div id = "div2"> DIV2 </div> <div id = "div3"> DIV3 </div> </div> <script> function setBottom() { const root = document.getElementById('div1') const input_field = document.getElementById('input_field') root.style.perspectiveOrigin = input_field.value } </script> </body> </html>
In this tutorial, we learned how to set the bottom position of 3D elements with JavaScript. We have seen two examples where both of them use the style.perspectiveOrigin property. In the first example, we see how to set the bottom of a 3D element using a button click event. In the second example, we take the property value of style.perspectiveOrigin property from an input field, which is used to take the user’s desired value to set in the property. Users now have a proper understanding of how to set the bottom position of 3D elements.