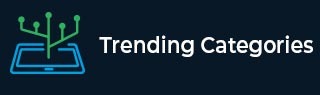
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to round up a number in JavaScript?
In this tutorial, we will discuss how to round up a number in JavaScript. There is a property in JavaScript under ‘Math’ named “ceil” with the help of this property we can easily round up a number in JavaScript. Ceil() is a mathematical function that computes an integer x to greater than or equal to x. If x has the decimal value zero or is a simple integer then it returns x, and if the number is a decimal number (x.yz, here yz is a non-zero number) then it returns x+1.
Syntax
var x = A.B var value = Math.ceil(x);
In the above syntax, first, we create variable ‘x’ which contains the value “A.B” after that we create another variable ‘value’ in which we assign integer x by applying the Math.ceil property to round up a number.
Algorithm
We have seen the syntax to round up a number, let’s see the complete algorithm step by step to understand it in a better way −
Step-1 − First, we need a variable that will be a real number and may contain some decimal part or may not contain a decimal part.
Step-2 − Later we are going to use Math.ceil() property on the current number.
Step-3 − Math.ceil() function will return an integer, and the return value does not contain any decimal part.
Step-4 − If the number passed to Math.ceil() function is a proper integer means its decimal part is zero then it will return the same number.
Step-5 − If the number passed to Math.ceil() function is a number with a decimal part non-zero then the function will make its decimal part equal to zero and return the next number to the remaining number.
We have seen some of the basic steps for how to round up a number in javascript, now let’s see some examples to get a better understanding of the above-defined steps.
Example 1
In this example, we are going to round up a number using the syntax defined above.
<html> <head> <title>JavaScript Math ceil() Method</title> </head> <body> <script> var x = 4; var value1 = Math.ceil( x ); document.write("First Test Value: " + value1 ); var y = 30.2; var value2 = Math.ceil( y ); document.write("<br />Second Test Value: " + value2 ); var z = 2.7; var value3 = Math.ceil( z ); document.write("<br />Second Test Value: " + value3 ); </script> </body> </html>
In the above example, we have applied the Math.ceil() property to three different variables x, y, and, z. First, we create the variable ‘x’ it is an integer so when we apply the Math.ceil property to it and assign that value to variable ‘value1’ then we print that value with the help of the document.write and it returns x.
Similarly, we have done the same with variables y and z. They both are decimal numbers so they return y+1 and z+1 respectively after applying the property Math.ceil to them.
Note − Similar to the ceil() function we have floor() function. As the name suggests ceil means the above the floor() means the ground and it decreases a number. If the given number is a perfect integer with zero decimal part then as ceil() function, floor() function doesn't do any operation but if the decimal part is non-zero for the number passed as the parameter, then floor() function will remove the decimal part and will return the remaining integer part.
Example 2
<html> <head> <title>JavaScript Math floor() Method</title> </head> <body> <script> var x = 4; var value1 = Math.floor( x ); document.write("First Test Value: " + value1 ); var y = 30.2; var value2 = Math.floor( y ); document.write("<br />Second Test Value: " + value2 ); var z = 2.7; var value3 = Math.floor( z ); document.write("<br />Second Test Value: " + value3 ); </script> </body> </html>
In the above example, we have applied the Math.floor() property to three different variables x, y, and, z. First, we create the variable ‘x’ it is an integer so when we apply the Math.floor property to it and assign that value to variable ‘value1’ then we print that value with the help of the document.write and it returns x.
Conclusion
In this tutorial, we have learned how to round up a number in JavaScript. There is a property in JavaScript named “Math.ceil” with the help of this property we can easily round up a number. There are basically two types of numbers that we need to round up, they are decimal numbers and non-decimal numbers.
In decimal numbers the property returns plus one to the original number and in non-decimal numbers it returns the same as the actual number.