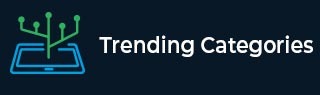
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to print date in a regular format in Python?
If you print the dates directly using a print function, you'd get regular dates,
Example
import datetime today = datetime.date.today() print(today)
Output
You will get the output −
2018-1-2
which is exactly what you want. But when you append this to a list and then try to print it,
Example
import datetime my_list = [] today = datetime.date.today() my_list.append(today) print(my_list)
Output
You will get the output −
[datetime.date(2018, 1, 2)]
This is happening because datetimes are objects. Therefore, when you manipulate them, you manipulate objects, not strings, not timestamps nor anything. Any object in Python have TWO string representations. The regular representation that is used by "print", can be get using the str() function. This one is implemented using the __str__ function in the class for that object. The alternative representation that is used to represent the object nature (as a data). It can be get using the repr() function.
So in order to get the correct representation, you need to call str explicitly on your datetime objects.
Example
import datetime my_list = [] today = datetime.date.today() my_list.append(str(today)) print(my_list)
Output
You will get the output −
['2018-01-02']