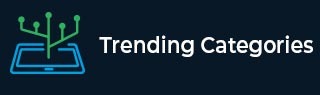
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to parse a string to an int in C++?
You can use a string stream to parse an int in c++ to an int. You need to do some error checking in this method.
example
#include<iostream> #include<sstream> using namespace std; int str_to_int(const string &str) { stringstream ss(str); int num; ss >> num; return num; } int main() { string s = "12345"; int x = str_to_int(s); cout << x; }
Output
This will give the output −
12345
In the new C++11, there are functions for that: stoi(string to int), stol(string to long), stoll(string to long long), stoul(string to unsigned long), etc.
Example
You can use these functions as follows −
#include<iostream> using namespace std; int main() { string s = "12345"; int x = stoi(s); cout << x; }
Output
This will give the output −
12345
Advertisements