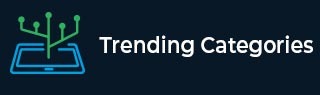
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to organize Python classes in modules and/or packages
There are different modules or packages in the python classes. When we use their names as it is in the code it will be somewhat clumsy and not good to see. So, we need to organize the python classes in modules and packages.
Modules are the group of functions, classes or any block of code kept in a single file. The file extension of the methods will be .py.
If the python code is with 300-400 lines of code, then it can be made as a module for better understandability.
The module name can be available as a global variable. So that we can access the module anytime in the code as per the requirement as it will become the global variable.
Modules contain the executable code or functions. If we want to use the module in our code, we can import them using the import method available in python.
The packages are the group of multiple modules in a separate directory. These can be said as folders with modules and init.py file.
The organizing of python classes in modules and packages depends upon our personal preferences, interest and application.
So that’s the reason each class will be kept in separate files. In some cases, the similar classes will be placed in the same file as per the application requirement, scenario etc. We can say that the classes are organized into modules and packages depending upon the requirement.
Example
Let’s see an example to organize the packages and modules of the class in python. The following is the code.
To get the current date, we import the Python module DateTime as an alias (dt) for convenience. Within this module are several methods, including 'date', which in turn has a function today(). We use all of these together and assign their output to a variable named 'tday' before printing out the result.
import datetime as dt today = dt.date.today() print("Current date is:",today)
Output
The following is the output of the organizing of the packages and modules.
Current date is: 2022-09-20
Example
In the previous example we imported the datetime module alone. Now, let's see another example to organize the packages and modules of the class in python by importing the method along with the module name.
from datetime import date as d today = d.today() print("Current date is:",today)
Output
Current date is: 2022-09-20
Example
The numpy library is a collection of packages and modules which can be used as per our requirement. In this example we will see about the organizing of numpy library packages and modules.
import numpy as np a = np.array([12,3,4,5,6]) print("Created array:",a)
Output
The following is the output of the organizing of the packages and modules.
Created array: [12 3 4 5 6]
Example
Let’s see another example to understand the organizing of the numpy library modules and packages.
import numpy as np a = np.array([[12,3,4,5,6],[20,2,4,0,3]]) print("Created array:",a)
Output
Created array: [[12 3 4 5 6] [20 2 4 0 3]]