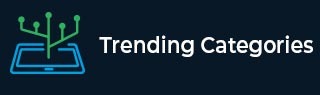
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to initialize a boolean array in JavaScript?
In this tutorial, we will learn how to initialize a boolean array in JavaScript.
We can initialize a boolean array in JavaScript in three ways−
Using the fill() Method
Using the Array from() Method
Using the for Loop
Using the fill() Method
We can initialize a boolean array using the fill() method. The fill() method sets the static value of all array elements from the beginning index to the end index.
Syntax
//initialization of boolean array with fill() method let arrayName = new Array(array_length).fill(true/false);
In the above syntax, we create an array with the name "arrayName" and initialize an Array() constructor with the length of the array, then call the fill() method of the array to initialize all the values with true or false.
Example
In the example below, we learned how users could initialize a boolean array in JavaScript using the fill() method. Here we used a "Click Here" button with a click event. After clicking that button, the bool() function is invoked. There we create an array "myarray" and initialize it with the Array.fill() method and set the value to true.
<html> <body> <h3>Initializing a boolean array using fill() method</h3> <p>Boolean array with all true values</p> <button onclick="bool()">Click Here</button> <div id="root"></div> <script> function bool() { //initializing an array named "myarray" with a length of 5 const myarray = new Array(5).fill(true) document.getElementById('root').innerHTML = "[" + myarray + "]" } </script> </body> </html>
Users can see that after clicking the button, the div tag with id "root" shows the array filled with true values.
Using the Array from() Method
Using the Array.from() method, we can initialize a boolean array. The Array.from() method accepts a length parameter and returns an array from any iterable or object.
Syntax
// initialization of boolean array with Array.from() method let arrName = Array.from({length}, (value,index)=> true/false);
In the above syntax, we create an array with the name "arrName" and initialize an Array.from() method with the array length and set the value as true or false in all index elements.
Example
In the example below, we learned how users could initialize a boolean array in JavaScript using the Array.from() method. Here we used a "Click Here" button with a click event. After clicking that button, the bool() function is invoked. There we create an array "myarray" and initialize it with the Array.from() method with a length of 5 and a value initialized with false.
<html> <body> <h3>Initializing a boolean array using Array.from() method</h3> <p>Boolean array with all false values</p> <button onclick="bool()">Click Here</button> <div id="root"></div> <script> function bool() { //initializing an array named "myarray" with a length of 5 const myarray = Array.from({ length: 5 }, (value, index) => false); document.getElementById('root').innerHTML = "[" + myarray + "]" } </script> </body> </html>
Users can see that after clicking the button, the div tag with id "root" shows the array filled with false values.
Using the for Loop
We can initialize a boolean array using a for loop also. First, we have to initialize an array, arrName, with a blank value. Then, as for loop work, we first initialize a variable and increase its value under a condition. If the condition is true, we will push the boolean value into the array with the push() method.
Syntax
// initialization of boolean array with for loop Let arrName = [] for (expression 1; expression 2; expression 3){ arrName.push(true/false); }
In the above syntax, we create an array with the name "arrName" and initialize it as an empty array. Then, the boolean value is pushed into the arrName array using the for loop iteration method.
Example
In the example below, we learned how users could initialize a boolean array in JavaScript using the for loop iteration. Here we used a "Click Here" button with an onclick event handler function. After clicking that button, the bool() function is invoked. There we create an array "myarray" and create a for loop in the range of 1 to 5. If the array position is even, we insert a true value in an even position and a false value in an odd position. We are setting the values of the elements using the push() method.
<html> <body> <h3>Initializing a boolean array using for loop</h3> <p>Boolean array with true values in even positions and false values in odd positions</p> <button onclick= "bool()">Click Here</button> <div id="root"></div> <script> function bool() { //initializing an array named "myarray" with a length of 5 let myarray = [] for (let i = 0; i < 5; i++) { if (i % 2 == 0) { myarray.push(true); } else { myarray.push(false); } } document.getElementById('root').innerHTML = "[" + myarray + "]" } </script> </body> </html>
Users can see that after clicking the button, the div tag with id "root" shows the array filled with true and false values with their positions, respectively.
In this tutorial, we learned how we could initialize a Boolean array in two different approaches. The first two approaches can only initialize a static Boolean value for all of the array elements, but in the for loop iteration approach, we can set our custom logic for initializing the values of a boolean array.