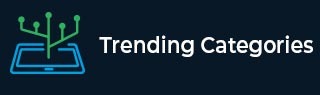
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to get the number of options in the dropdown list with JavaScript?
This tutorial will show different ways to get the number of options in the drop-down list with JavaScript.
While filling out any form online, users have to add unique information using the input method. However, the options they get in the drop-down list are already determined. Since there can be limited options mentioned in the drop-down, you might have to check the number using JavaScript.
Using options.length method to get the number of drop-down options
One of the simple ways to get access to the list of options is to get the list by its Id. We will use a document.getElementById() method to select the list. Then, by using the .options.length method, we will get the number of the drop-down list.
Syntax
In this syntax, we will use the id of the <select> tag and .options.length method to determine how many options have been created in the drop-down list.
var list = document.getElementById("dropdown"); drop.innerHTML = list.options.length;
Algorithm
Step 1 − Create a drop-down list using <select> tag in the document.
Step 2 − Add 2-3 options in the list with <option> tag, as shown in example.
Step 3 − In JavaScript, use document.getElementById() method to get access to the <select> tag.
Step 4 − Use .options.length and innerHTML to get the number of options created in drop-down list.
Example
Check the example below to understand the best way to create a drop-down list in an HTML document. We got the number of options created using the id of <select> tag and .options.length method.
<html> <body> <h2>Using <i>options.length method</i> to get the number of options</h2> <select id = "dropdown"> <option>French</option> <option>English</option> <option>Español</option> </select> <p id = numdrop></p> </body> <script> var list = document.getElementById("dropdown"); let drop = list.options.length + " options"; document.getElementById('numdrop').innerHTML = drop; </script> </html>
Using .querySelectorAll() method to the number of drop-down options
Since the options are created using the <options> tag within the document, you can use the .querySelectorAll() method to access the length as well as the value.
Syntax
We will follow the below mention syntax to get the number of options added to the drop-down list.
drop.innerHTML = document.querySelectorAll('option').length;
Example
Here we didn’t create any id for the <select> tag. We will use the querySelectorAll() method in JavaScript to get the number of options. Then, simply get the output with the .length and innerHTML method.
<html> <body> <h2>Using <i>.querySelectorAll() method</i> to get the number of options</h2> <select> <option>French</option> <option>English</option> <option>Español</option> </select> <p id = num></p> </body> <script> var drop = document.querySelectorAll('option').length; document.getElementById('num').innerHTML = drop; </script> </html>
Using Increment Operators to get the number of drop-down options
Using increment operators to get the number of options is most common because it allows you to make changes in the output. You can check the example to understand how the increment method can give desired output with several drop-down options as well as the text.
Syntax
In this syntax, we have got the <select> tag using its id. Then, we got the number of drop-down options and the text using increment operators. You can change the output by changing the value of i from 0 to 1 or 2.
a = document.getElementById("dropdown"); options = ""; for (i = 0; i < a.length; i++) { options = options + a.options[i].text;
Example
Here we have got the number of drop-down options and the option’s text using increment operators in JavaScript.
<html> <body> <h2>Using <i>Increment Operators</i> to get the No. of options</h2> <select id = "list"> <option>French</option> <option>English</option> <option>Español</option> </select> <p id = num></p> </body> <script> var a, i, options; a = document.getElementById("list"); options = ""; for (i = 0; i < a.length; i++) { options = options + "<br> " + a.options[i].text; } document.getElementById('num').innerHTML = a.length + " Dropdown Options: " + options; </script> </html>
Although getting the number of options using the .length method can give the output instantly, you can use increment operators to customize the output in JavaScript. For instance, if you want to check the options that were added recently from the drop-down list that include more than 100 options, you can change the value of i to get the list.