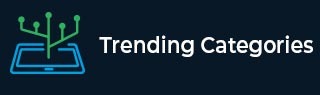
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to get objects by ID, Class, Tag, and Attribute in jQuery?
Here’s how you can get objects by ID Selector (#id), by Class Selector (.class), by Tag, and Attribute (.attr()).
Get Object by Class Selector
Example
The element class selector selects all the elements which match with the given class of the elements.
<html> <head> <title>jQuery Selector</title> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <script> $(document).ready(function() { $(".big").css("background-color", "yellow"); }); </script> </head> <body> <div class = "big" id="div1"> <p>This is first division of the DOM.</p> </div> <div class = "medium" id = "div2"> <p>This is second division of the DOM.</p> </div> </body> </html>
Get Object by ID Selector
Example
The element ID selector selects a single element with the given id attribute:
<html> <head> <title>jQuery Selector</title> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <script> $(function(){ $("#submit").click(function(){ alert($('input:radio:checked').val()); }); }); </script> </head> <body> <form id="myForm"> Select a number:<br> <input type="radio" name="q1" value="1">1 <input type="radio" name="q1" value="2">2 <input type="radio" name="q1" value="3">3<br> <button id="submit">Result</button> </form> </body> </html>
Get Object by Tag
Example
For this, pass the name of the specific tag i.e. <a> tag below:
<html> <head> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <script> $(document).ready(function(){ $("a").click(function(){ $("a.active").removeClass("active"); $(this).addClass("active"); }); }); </script> <style> .active { font-size: 22px; } </style> </head> <body> <a href="#" class="">One</a> <a href="#" class="">Two</a> <p>Click any of the link above and you can see the changes.</p> </body> </html>
Get Object by Attribute
Example
Using the .attr(), you can get any attribute of any tag. Here’s an example showing how to get an attribute value:
<html> <head> <title>jQuery Example</title> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <script> $(document).ready(function(){ $("button").click(function(){ $("img").attr("height", "200"); }); }); </script> </head> <body> <img src="/green/images/logo.png" alt="logo" width="450" height="160"><br> <button>Change the height</button> </body> </html>
Advertisements