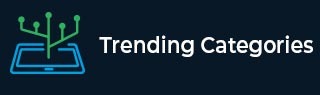
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to find the index of an item in a C# list in a single step?
To get the index of an item in a single line, use the FindIndex() and Contains() methods.
int index = myList.FindIndex(a => a.Contains("Tennis"));
Above, we got the index of an element using the FindIndex(), which is assisted by Contains() method for that specific element.
Here is the complete code −
Example
using System; using System.Collections.Generic; public class Program { public static void Main() { List < string > myList = new List < string > () { "Football", "Soccer", "Tennis", }; // finding index int index = myList.FindIndex(a => a.Contains("Tennis")); // displaying index Console.WriteLine("List Item Tennis Found at index: " + index); } }
Output
List Item Tennis Found at index: 2
Advertisements