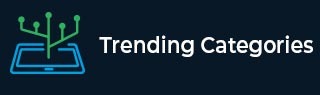
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to find a character, not between the brackets in JavaScript RegExp?
RegExp or RegEx in JavaScript is the short form of Regular Expressions. Regular Expressions are used to check validity of a particular combination of characters, digits, or any other symbol entered by the user. Regular expressions are mainly used for checking valid emails, and passwords that authenticate the correct user to log in.
In this tutorial, we will learn how we can find a character that is not present between the brackets of JavaScript RegExp.
Syntax
Following is the syntax that is used to find the character, not between the brackets of JavaScript RegExp −
[^……]
In above syntax, a square bracket is used in the basic syntax of RegExp. While the symbol ^ is used to check whether the character or string after the symbol is present in the sentence or not, and the dots are used to indicate the character that you are searching for.
Following is the list of the items that you can check whether they are present in the sentence or not −
- [^a] − It will check for the absence of only a in the sentence.
- [^abc] − It will check for the absence of each character individually (a, b, c) as well as combined (abc) in the sentence.
- [^A-Z] − It will check for not any character in the range from uppercase A to uppercase Z present in the sentence.
- [^a-z] − It will check for not any character in the range from lowercase a to lowercase z present in the sentence.
- [^A-z] − It will check for not any character in the range from uppercase A to lowercase z present in the sentence.
Let us discuss all of them with code examples −
Algorithm
STEP 1 − In the first step of the algorithm, you need to define a sentence in which you will check for the character, not between the brackets of JavaScript RegExp.
STEP 2 − In second step, you will define the regular expression for a particular character or combination of characters that you will check for, according to the syntax discussed above.
STEP 3 − In next step, you will use the in-built match() function or method of JavaScript to match the original sentence with the regular expression defined by you.
STEP 4 − In the last or the fourth step, you will display the sentence obtained after matching the original sentence with regular expression.
Example 1
Below example will explain how we can find a single character that is not in the brackets of JavaScript RegExp.
<!DOCTYPE html> <html> <head> <title>Example - find a character, not between the brackets in JavaScript RegExp</title></head> <body> <h3>Find a character, not between the brackets in JavaScript RegEx</h3> <p>It will do a global search for the characters that are not "t":</p> <p>Original Sentence: Hey there, we are checking for character, not between the brackets of JavaScript RegExp.</p> <button onclick="display()">Check for character</button> <p id="result"></p> <script> function display() { let myStr = "Hey there, we are checking for character, not between the brackets of JavaScript RegExp."; let reg = /[^t]/g; let match = myStr.match(reg); document.getElementById("result").innerHTML = match; } </script> </body> </html>
In above example, we have search for the character t that is not present in the backets of the JavaScript RegExp. Hence, It matches the RegExp from the original sentence and removes every occurrence of the character passed, in this case t will be removed from the whole sentence.
Example 2
Below example will illustrate how we can find characters between a particular range (az and A-Z) that are not in the brackets of JavaScript RegExp.
<!DOCTYPE html> <html> <body> <h3>Find a character, not between the brackets in JavaScript RegExp</h3> <p>It will do a global search for the characters that are not in range from "a-z" and "A-Z":</p> <p>Original Sentence: <b>Hey there, we are checking for character, not between the brackets of JavaScript RegExp.</b></p> <button onclick="display()">Check for character(in range a to z)</button> <button onclick="display2()">Check for character(in range A to Z)</button> <p id="result"></p> <script> function display() { let myStr = "Hey there, we are checking for character, not between the brackets of JavaScript RegExp."; let reg = /[^a-z]/g; let match = myStr.match(reg); document.getElementById("result").innerHTML = match; } function display2() { let myStr = "Hey there, we are checking for character, not between the brackets of JavaScript RegExp."; let reg = /[^A-Z]/g; let match = myStr.match(reg); document.getElementById("result").innerHTML = match; } </script> </body> </html>
In the above example, we are checking for the character not in the range from lowercase a to lowercase z and from uppercase A to uppercase Z. If you click the button Check for character(in range a to z) then it will remove all the lowercase characters from the original sentence. Similarly, if you click Check for character(in range A to Z) then it will remove all the uppercase letters from the original sentence.
Example 3
Below example will explain how we can check for the different characters individually as well as the combination of those characters at the same time that is not in the brackets of JavaScript RegExp −
<!DOCTYPE html> <html> <body> <h3>Find a character, not between the brackets in JavaScript RegExp</h3> <p>It will do a global search for the characters as well as combination of characters except chareacters in "there"</p> <p>Original Sentence: Hey there, we are checking for character, not between the brackets of JavaScript RegExp.</p> <button onclick="display()">Check for character</button> <p id="result"></p> <script> function display() { let myStr = "Hey there, we are checking for character, not between the brackets of JavaScript RegExp."; let reg = /[^there]/g; let match = myStr.match(reg); document.getElementById("result").innerHTML = match; } </script> </body> </html>
In the above example, we can clearly see that there is removed from the original sentence as well as the characters t, h, e, r, and e are also removed individually.
In this tutorial, we have seen how we can find a character, not between the brackets of JavaScript RegExp with three different examples for checking different characters or words. These examples will help you to check whether any character according to your given criteria is present in a particular input field like email, password, or not.