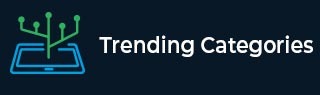
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to execute a Python file in Python shell?
Venturing further into the realm of Python programming, you'll undoubtedly encounter scenarios where executing a Python file from within the Python shell becomes essential. This capability endows you with the power to test, run, and interact with your Python scripts seamlessly without exiting the Python environment. Within this article, we shall discuss some distinct methods to execute Python files within the Python shell, each offering its unique functionalities and adaptability, facilitating seamless interaction with your Python scripts. As a Python coding expert, I would walk you through each method with step-by-step explanations and examples in a lucid style that is user-friendly. By the conclusion of this article, you shall gain the strategies to execute Python files within the Python shell akin to a seasoned Python developer. So let us take a plunge into the examples and intricacies of working with the Python shell!
A Glimpse into the Python Shell and Execution
Before we delve into the code examples, let us briefly acquaint ourselves with the Python shell and its profound role in executing Python files. The Python shell, often referred to as the interactive interpreter, represents a formidable tool that facilitates the execution of Python commands and scripts interactively. Executing a Python file within the Python shell empowers you to scrutinize specific segments of your script, interact with its functions, and inspect variables in real time.
Harnessing the %run Magic Command in IPython
Our first approach unveils the execution of a Python file in IPython using the %run magic command.
Launch the IPython shell:
ipython
If your_script.py is
print('Hello World!')
Execute the Python file your_script.py using %run:
Example
%run your_script.py
Output
Hello World!
In this illustration, we initiate the IPython shell using the ipython command. Inside the shell, we execute the Python file your_script.py with the %run magic command. This method grants you the capability to execute Python files seamlessly from within the IPython environment.
Leveraging the exec() Function
Our second method demonstrates Python file execution using the exec() function.
Example
with open("your_script.py", "r") as file: script_code = file.read() exec(script_code)
Output
Hello World!
Here, we access the Python file your_script.py in read mode using the open() function, saving its contents within the script_code variable. Subsequently, we utilize the exec() function to execute the Python code present in script_code. This methodology bestows greater control over the execution environment and is ideal for executing Python code from a file.
Employing subprocess.run()
The third method exhibits Python file execution via the subprocess.run() function.
Example
import subprocess subprocess.run(["python", "your_script.py"])
Output
Hello World! CompletedProcess(args=['python', '\content\your_script.py'], returncode=2)
In this instance, we import the subprocess module, providing capabilities to spawn new processes, connect to their input/output/error pipes, and retrieve their return codes. We proceed to use the subprocess.run() function to execute the Python file your_script.py, passing the Python interpreter as an argument. This method proves advantageous when you aim to execute the Python file as a separate, independent process.
Executing with execfile() (Python 2 only)
Our fourth method demonstrates Python file execution using the execfile() function. It's crucial to note that this method is exclusive to Python 2 and not available in Python 3.
Example
execfile("your_script.py")
Output
Hello World!
In this example, we employ the execfile() function to execute the Python file your_script.py directly. Do keep in mind that this method is specific to Python 2 and should solely be utilized in Python 2 environments.
Embracing the importlib module
Our final approach showcases Python file execution through the importlib module, granting enhanced control and flexibility.
Example
import importlib.util file_path = "your_script.py" module_name = "your_script_module" spec = importlib.util.spec_from_file_location(module_name, file_path) module = importlib.util.module_from_spec(spec) spec.loader.exec_module(module)
Output
Hello World!
Here, we import the importlib.util module, which furnishes functions for working with modules. Defining the file_path variable to retain the Python file's path and the module_name variable to designate the desired module name, we proceed to create a module specification using importlib.util.spec_from_file_location() and a module object using importlib.util.module_from_spec(). Finally, we execute the Python file by invoking spec.loader.exec_module(module). This approach proves exceptionally valuable when you aim to load and execute Python files dynamically.
Executing Python files within the Python shell stands as a priceless skill that simplifies code testing, debugging, and interaction. Throughout this enlightening article, we delved into a few different methods to execute Python files, each offering unique advantages catered to your individual needs and workflow.
Be it the %run magic command in IPython, the exec() function, subprocess.run(), execfile() (Python 2 only), or the importlib module, each method bestows upon you the ability to interact with your Python scripts and explore their functionalities with finesse.
As your Python journey continues, embrace the potency of executing Python files within the Python shell, elevating your coding experience, and mastering the art of interactive Python development. May the Python shell stand as your infallible tool in testing and delving into the realm of your Python scripts!