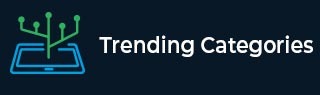
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to differentiate between Manual and Automated Animation in JavaScript?
Generally, animation in JavaScript is done to get different effects and make the object move around the page. You can move and animate any type of HTML element using manual or automated animations in JavaScript.
In this tutorial, we will learn how to differentiate between manual and automated animation in JavaScript.
Manual Animation
Before explaining the difference between the two common types of animation used in JavaScript, we must learn the process of animating objects manually.
Under Manual Animation, the animation process is not automated. The following is the implementation of a simple animation using DOM object properties and JavaScript functions as follows. The following list contains different DOM methods.
We are using the JavaScript function getElementById to get a DOM object and then assigning it to a global variable imgObj
We have defined an initialization function init() to initialize imgObj where we have set its position and left attributes
We are calling the initialization function at the time of window load.
Finally, we are calling moveRight() function to increase the left distance by 10 pixels. You could also set it to a negative value to move it to the left side.
Steps
Step 1 − Choose or decide the object or image that needs to be animated manually by the user.
Step 2 − Decide the position and size of the elements.
Step 3 − Add a button or another element that can be used with the onClick method to manually animate the object.
Step 4 − Determine the movement or change in the object with every click.
Example
The following example shows how to create objects or add images that can be moved or animated manually using the moveRight() or moveLeft() functions.
<html> <head> <title>JavaScript Manual Animation</title> <script> var imgObj = null; function init() { imgObj = document.getElementById('myImage'); imgObj.style.position= 'relative'; imgObj.style.left = '0px'; } function moveRight() { imgObj.style.left = parseInt(imgObj.style.left) + 10 +'px'; } window.onload =init; </script> </head> <body> <form> <img id="myImage" src="https://www.tutorialspoint.com/javascript/images/javascript-mini-logo.jpg" /> <p>Click button below to move the image to right</p> <input type = "button" value = "Click Me" onclick = "moveRight();" /> </form> </body> </html>
Automated animation
Automated animation creates better and more user-friendly software and online games. The main benefit of using automated animation is setting the time for certain effects or movements of the objects.
We will automate the process discussed in the manual animation. We can automate this process by using the JavaScript function setTimeout() as follows −
Here we have added more methods. So let's see what is new here −
The moveRight() function is calling setTimeout() function to set the position of imgObj.
- We have added a new function stop() to clear the timer set by setTimeout() function and to set the object at its initial position.
Steps
Step 1 − Find or create the object that will be used for automated animation.
Step 2 − Choose the initial style and position of the object..
Step 3 − Add a button, if required, to start and stop the animation process.
Step 4 − Determine the time or position where the animation needs to stop or restart.
Example
You can see from the following example that an object can stop animation automatically at a certain point. This example can be used to experiment and find out the scope of automated animation in JavaScript
<html> <head> <title>JavaScript Automated Animation</title> <script> var imgObj = null; var animate ; function init() { imgObj = document.getElementById('myImage'); imgObj.style.position= 'relative'; imgObj.style.left = '0px'; } function moveRight() { imgObj.style.left = parseInt(imgObj.style.left) + 10 + 'px'; animate = setTimeout(moveRight,20); // call moveRight in 20msec } function stop(){ clearTimeout(animate); imgObj.style.left = '0px'; } window.onload =init; </script> </head> <body> <form> <img id="myImage" src="https://www.tutorialspoint.com/javascript/images/javascript-mini-logo.jpg" /> <p>Click the buttons below to handle animation</p> <input type = "button" value = "Start" onclick = "moveRight();" /> <input type = "button" value = "Stop" onclick = "stop();" /> </form> </body> </html>
How do we differentiate between manual and automated animation?
Step 1 − Find out if you have to make manual changes to get the results.
For instance, if you want to subscribe to a website, you can either see an automated pop-up subscription form, or you have to go click a button to see the form.
Step 2 − Check the time limit of the animation.
In manual animation, you won’t see the animation working without clicking a button. Generally, in automated animation, the fade-in or other functionalities can continue.
JavaScript Animation with the movement of the mouse
You can see various animations with the movement of the mouse. While creating responsive buttons, images, and other elements, we commonly use JavaScript. In this method, you will see how the image size changes with the movement of the mouse over the element.
Syntax
The following syntax shows how to change the elements using onMoveOver and onMoveOut user behaviors.
if(document.images){ var animation1 = new Image(); var animation2 = new Image(); } onMouseOver = animation2.src; onMouseOut = animation1.src;
Example
The following example shows how an object can change its size or position with the movement of the mouse over the element.
<html> <head> <script> if(document.images){ var animation1 = new Image(); // Preload an image animation1.src = "https://demo.sirv.com/nuphar.jpg?w=400&h=250"; var animation2 = new Image(); // Preload second image animation2.src = "https://demo.sirv.com/nuphar.jpg?w=200&h=450"; } </script> </head> <body> <h3> Change in image size with the <i> movement of a mouse </i>. </h3> <a id = SVG href = "#" onMouseOver = "document.myImage.src=animation2.src;" onMouseOut = "document.myImage.src = animation1.src;"> <img id = SVG name = "myImage" src = "https://demo.sirv.com/nuphar.jpg?w=400&h=250" /> </a> </body> </html>
Using the above method, you can find the number of arguments that have been passed within a JavaScript function. You will see the output of the result by running this code.