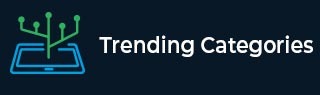
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to destroy an object in Python?
When an object is deleted or destroyed, a destructor is invoked. Before terminating an object, cleanup tasks like closing database connections or filehandles are completed using the destructor.
The garbage collector in Python manages memory automatically. for instance, when an object is no longer relevant, it clears the memory.
In Python, the destructor is entirely automatic and never called manually. In the following two scenarios, the destructor is called −
- When an object is no longer relevant or it goes out of scope
- The object's reference counter reaches zero.
Using the __del__() method
In Python, a destructor is defined using the specific function __del__(). For instance, when we run del object name, the object's destructor is automatically called, and it then gets garbage collected.
Example 1
Following is an example of destructor using __del__() method −
# creating a class named destructor class destructor: # initializing the class def __init__(self): print ("Object gets created"); # calling the destructor def __del__(self): print ("Object gets destroyed"); # create an object Object = destructor(); # deleting the object del Object;
Output
Following is an output of the above code −
Object gets created Object gets destroyed
Note − The destructor in the code above is invoked either after the program has finished running or when references to the object are deleted. This indicates that the object's reference count drops to zero now rather than when it leaves the scope.
Example 2
In the following example, we will use Python's del keyword for destroying user-defined objects −
class destructor: Numbers = 10 def formatNumbers(self): return "@" + str(Numbers) del destructor print(destructor)
Output
Following is an output of the above code −
NameError: name 'destructor' is not defined
We get the above error as ‘destructor’ gets destroyed.
Example 3
In the following example, we will see −
- How to use the destructor
- When we delete the object, how the destructor is called?
class destructor: # using constructor def __init__(self, name): print('Inside the Constructor') self.name = name print('Object gets initialized') def show(self): print('The name is', self.name) # using destructor def __del__(self): print('Inside the destructor') print('Object gets destroyed') # creating an object d = destructor('Destroyed') d.show() # deleting the object del d
Output
We made an object using the code above. A reference variable called d is used to identify the newly generated object. When the reference to the object is destroyed or its reference count is 0, the destructor has been called.
Inside the Constructor Object gets initialized The name is Destroyed Inside the destructor Object gets destroyed
Things to Keep in Mind About Destructor
- When an object's reference count reaches 0, the __del__ method is invoked for that object.
- When the application closes or we manually remove all references using the del keyword, the reference count for that object drops to zero.
- When we delete an object reference, the destructor won't be called. It won't run until all references to the objects are removed.
Example
Let's use the example to grasp the above mentioned principles −
- Using d = destructor("Destroyed"), first create an object for the student class.
- Next, give object reference d to new object d1, using d1=d
- Now, the same object is referenced by both d and d1 variables.
- After that, we removed reference d.
- In order to understand that destructors only functions when all references to the object are destroyed, we added a 10 second sleep period to the main thread.
import time class destructor: # using constructor def __init__(self, name): print('Inside the Constructor') self.name = name print('Object gets initialized') def show(self): print('The name is', self.name) # using destructor def __del__(self): print('Inside the destructor') print('Object gets destroyed') # creating an object d = destructor('Destroyed') # Creating a new reference where both the reference points to same object d1=d d.show() # deleting the object d del d # adding sleep and observing the output time.sleep(10) print('After sleeping for 10 seconds') d1.show()
Output
Destructors are only called, as you can see in the output, after all references to the objects are removed.
Additionally, the destructor is called once the program has finished running and the object is ready for the garbage collector. (That is, we didn't explicitly use del d1 to remove object reference d1).
Inside the Constructor Object gets initialized The name is Destroyed After sleeping for 10 seconds The name is Destroyed