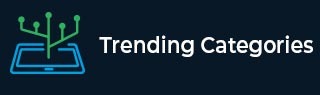
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to compare two different files line by line in Python?
This tutorial looks at various Python comparison techniques for two files. We'll go over how to perform this typical work by using the available modules, reading two files, and comparing them line by line.
In Python, comparing two files can be done in a variety of ways.
Comparing Two Text Files Line by Line
Using the open() function to read the data from two text files, we can compare the information they contain. The local directory will be searched for and potentially read by the open() function.
Example
We'll contrast two files containing python data in this example. We're informed that these two python files might not be the same. Python will check the files for us. It is possible to extract the lines from the python file using the readlines() method.
file1 = open('D:\Work TP/moving files.py', 'r') file2 = open('D:\Work TP/mysql_access.py', 'r') File3 = open('D:\Work TP/trial.py', 'w') for line1 in file1: for line2 in file2: if line1 == line2: File3.write("%s\n" %(line1)) File3.close() file1.close() file2.close()
A for loop is used to compare the files line by line after the data has been extracted. The user is informed where the mismatch occurred if the lines don't match by a message. To make it simple for the user to locate the various lines, we will include the data itself.
Output
Following is an output of the above code -
Line 1 doesn't match. ------------------------ File1: # importing the modules File2: import mysql.connector Line 2 doesn't match. ------------------------ File1: import os File2: sampleDB=mysql.connector.connect(host='localhost',user='root',password='password',database='textx') Line 3 doesn't match. ------------------------ File1: import shutil File2: #print (sampleDB.connection_id) Line 4 doesn't match. ------------------------ File1: # Providing the folder path File2: cur=sampleDB.cursor() Line 5 doesn't match. ------------------------ File1: origin = 'C:\Users\Lenovo\Downloads\Works\' File2: s="CREATE TABLE novel(novelid integer(4),name varchar(20),price float(5,2))" Line 6 doesn't match. ------------------------ File1: target = 'C:\Users\Lenovo\Downloads\Work TP\' File2: cur.execute(s)
Using the filecmp Module
Work with files in Python is made possible via the filecmp module. This module is designed specifically to compare data between two or more files. Using the filecmp.cmp() method, we can accomplish this. If the files match, this function will return True; otherwise, it will return False.
Example
Following is an example using filecmpule −
import filecmp import os # notice the two backslashes File1 = "D:\Work TP\moving files.py" File2 = "D:\Work TP\trial.py" def compare_files(File1,File2): compare = filecmp.cmp(File1,File2) if compare == True: print("The two files are the same.") else: print("The two files are different.") compare_files(File1,File2)
Output
Following is an output of the above code −
The two files are different.
Using the difflib Module
For comparing texts and identifying variations between them, use the difflib package. The language is already pre-packaged with this Python 3 module. It has a lot of helpful features for comparing text samples.
First, we'll identify differences between two data files using the unified diff() function.We'll compare these files next.
Example1-Using unified-diff()
The with statement is used to read file data in the example that follows. The Python with statement allows us to open and read files securely −
import difflib with open("D:\Work TP/moving files.py",'r') as file1: file1_info = file1.readlines() with open("D:\Work TP/trial.py",'r') as file2: file2_info = file2.readlines() diff = difflib.unified_diff( file1_info, file2_info, fromfile="file1.py", tofile="file2.py", lineterm='') for lines in diff: print(lines)
Output
Following is an ouput of the above code −
--- file1.py +++ file2.py @@ -1,12 +0,0 @@ -# importing the modules -import os -import shutil -# Providing the folder path -origin = 'C:\Users\Lenovo\Downloads\Works\' -target = 'C:\Users\Lenovo\Downloads\Work TP\' -# Fetching the list of all the files -files = os.listdir(origin) -# Fetching all the files to directory -for file_name in files: - shutil.copy2(origin+file_name, target+file_name) -print("Files are copied succesfully")
Example2-Using differ()
Within the difflib library, there is a class called Differ that may be used to compare file differences. This class is used to compare groups of lines of text and generate deltas or differences.
Following is an example to compare two different files line-by-line using differ() method −
from difflib import Differ with open('D:\Work TP/moving files.py') as file1, open('D:\Work TP/trial.py') as file2: vary = Differ() for lines in vary.compare(file1.readlines(), file2.readlines()): print(lines)
Output
Following is an output of the above code −
- # importing the modules - import os - import shutil - # Providing the folder path - origin = 'C:\Users\Lenovo\Downloads\Works\' - target = 'C:\Users\Lenovo\Downloads\Work TP\' - # Fetching the list of all the files - files = os.listdir(origin) - # Fetching all the files to directory - for file_name in files: - shutil.copy2(origin+file_name, target+file_name) - print("Files are copied succesfully")