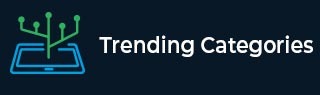
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to check if string or a substring of string ends with suffix in Python?
To check if a string or a substring of a string ends with a suffix in Python, there are several ways to accomplish this. Here are some examples:
Using the endswith() Method
The endswith() method checks if the string ends with a specified suffix and returns a boolean value. To check if a substring of a string ends with a suffix, you can use string slicing to get the substring and then apply endswith() on it.
Example
string = "hello world" suffix = "world" if string.endswith(suffix): print("String ends with suffix")
Output
String ends with suffix
Using the re Module
The re module provides support for regular expressions in Python. You can use regular expressions to match a pattern at the end of a string. Here's an example:
Example
import re string = "hello world" suffix = "world" pattern = re.compile(suffix + "$") if pattern.search(string): print("String ends with suffix")
Output
String ends with suffix
Using String Slicing
You can also use string slicing to check if a string or substring of a string ends with a suffix.
Example
string = "hello world" suffix = "world" if string[-len(suffix):] == suffix: print("String ends with suffix")
Output
String ends with suffix
To check if a string or a substring of a string ends with a specific suffix in Python, you can use the str.endswith() method. This method returns True if the string or substring ends with the specified suffix, and False otherwise.
Example
In this example, we define a string my_string and check if it ends with the specified suffix "aboard!". Since it does end with this suffix, the program prints "The string ends with 'aboard!'".
# Define a string my_string = "Welcome, aboard!" # Check if the string ends with "world!" if my_string.endswith("aboard!"): print("The string ends with 'aboard!'") else: print("The string does not end with 'aboard!'")
Output
The string ends with 'aboard!'
Example
In this example, we define a list of suffixes and a string my_string. We then use a generator expression with the any() function to check if my_string ends with any of the suffixes in suffixes. Since my_string ends with the suffix ".jpg", which is one of the suffixes in the list, the program prints "The string ends with one of the suffixes in the list".
# Define a list of suffixes suffixes = [".jpg", ".png", ".gif"] # Define a string my_string = "picture.jpg" # Check if the string ends with any of the suffixes if any(my_string.endswith(suffix) for suffix in suffixes): print("The string ends with one of the suffixes in the list") else: print("The string does not end with any of the suffixes in the list")
Output
The string ends with one of the suffixes in the list
Example
In this example, we check if the string my_string ends with the specified suffix "world". However, since my_string actually ends with "world!", the program prints "The string does not end with 'world'".
# Define a string my_string = "Hello, world!" # Check if the string ends with "world" if my_string.endswith("world"): print("The string ends with 'world'") else: print("The string does not end with 'world'")
Output
The string does not end with 'world'
Example
In this example, we check if the string my_string ends with any of the suffixes in the suffixes list. However, since my_string ends with ".txt", which is not one of the suffixes in the list, the program prints "The string does not end with any of the suffixes in the list".
# Define a list of suffixes suffixes = [".jpg", ".png", ".gif"] # Define a string my_string = "document.txt" # Check if the string ends with any of the suffixes if any(my_string.endswith(suffix) for suffix in suffixes): print("The string ends with one of the suffixes in the list") else: print("The string does not end with any of the suffixes in the list")
Output
The string does not end with any of the suffixes in the list