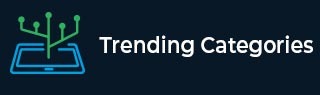
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to change the font size of a text using JavaScript?
In this tutorial, programmers will learn to change the font size of the text using JavaScript. Many application allows users to change the font size according to users’ requirement. We need to change the default font size using JavaScript to achieve that.
Before we move ahead with the tutorial, let’s learn what values we can assign to the fontsize.
Different values users can assign to the font size
We can assign the below values to the font size of any element. Every values changes the font size differently which we have explained here.
xx-large | x-large | large | medium | small | x-small | xx-small − Users can use these values to set fixed fonts size according to value.
smaller − It decreases the font size by single unit. If users are using the pixels for font size, it means ‘smaller’ decreases the font size by 1 px.
larger − It is the opposite of the smaller and increases the font size by one unit.
initial − It set the font size to default values which is the medium.
% − It set the font-size in percent to a parent element’s font size. For example, font size of the parent element is 100px, and we set 10% for child element, font size of child element will be 10px.
inherit − To set the font size of child as same as parent element’s font size.
So, we have seen different values which users can assign to the font size and users can use any of them according to their need.
Change the font size using JavaScript
In this section, we will learn to change the font size by accessing the HTML elements. Every HTML element contains the style attribute, and inside, it contains various styles for the element. We will access the element's font size using the style attribute and reassign a new value to it.
Users can follow the below syntax.
Syntax
let element = document.getElementById( "element_id" ); element.style.fontsize = "xx-large";
Example
In the below example, we will access the div element using the id and change the font size using JavaScript.
<html> <head> <style> button { height: 30px; width: 130px; } </style> </head> <body> <h2>Change the font size using JavaScript.</h2> <div>Click the button to change the font size using <i> font-size property of style attribute.</i></div> <div id = "fonts"> hello world!</div> <button onclick = "changeFontsize()" >change font size</button> <script> // function to change the font size of div function changeFontsize() { let fontsDiv = document.getElementById("fonts"); fontsDiv.style.fontSize = 'xx-large'; // change font size to xx-large } </script> </body> </html>
In the above output, users can see that when we click on the button, it changes the fontsize to xx-large.
Change the Font Size as the Parent Element’s Font Size
In this section, we will use the ‘inherit’ value to change the font size according to the parent element’s font size.
Syntax
let element = document.getElementById( "element_id" ); element.style.fontsize = “inherit”;
Example
In the below example, we have a child div inside the parent div. Initially, the font size of the text of the parent div is 50px, and the child div is 20px. When a user clicks on the button, it will set the font size of the child div to ‘inherit,’ which means the font size of the child div becomes the same as the parent div’s font size, which is 50px.
<html> <head> <style> button { height: 30px; width: 130px; } </style> </head> <body> <h2>Change the font size using JavaScript.</h2> <div style = "font-size: 50px;" > Click the button to change the font size <i> according to parent elements font size. </i> <div id = "fonts" style = "font-size:20px;" >hello world!</div> <button onclick = "changeFontsize()" >change font size</button> </div> <script> function changeFontsize() { let fontsDiv = document.getElementById("fonts"); fontsDiv.style.fontSize = 'inherit'; // change font size according to parent element's font size } </script> </body> </html>
Users can observe that, when they click on the button, font size of the “hello world!” text becomes same as its parent div’s font size.
In this tutorial, we have demonstrated how users can add functionality to change the font size using JavaScript. We have seen two various examples. In one example, we have set the fixed font size, ' xx-large’, and in another, we have set the ‘inherit’ value, which means it will inherit the font size from the parent element.
Also, we can set the smaller and larger value to the font size to decrease and increase it by a single unit.