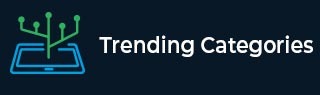
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to capture out of memory exception in C#?
The System.OutOfMemoryException occurs when the CLR fail in allocating enough memory that is needed.
System.OutOfMemoryException is inherited from the System.SystemException class.
Set the strings −
string StudentName = "Tom"; string StudentSubject = "Maths";
Now you need to initialize with allocated Capacity that is the length of initial value −
StringBuilder sBuilder = new StringBuilder(StudentName.Length, StudentName.Length);
Now, if you will try to insert additional value, the exception occurs.
sBuilder.Insert(value: StudentSubject, index: StudentName.Length - 1, count: 1);
The following exception occurs −
System.OutOfMemoryException: Out of memory
To capture the error, try the following code −
Example
using System; using System.Text; namespace Demo { class Program { static void Main(string[] args) { try { string StudentName = "Tom"; string StudentSubject = "Maths"; StringBuilder sBuilder = new StringBuilder(StudentName.Length, StudentName.Length); // Append initial value sBuilder.Append(StudentName); sBuilder.Insert(value: StudentSubject, index: StudentName.Length - 1, count: 1); } catch (System.OutOfMemoryException e) { Console.WriteLine("Error:"); Console.WriteLine(e); } } } }
The above handles OutOfMemoryException and generates the following error −
Output
Error: System.OutOfMemoryException: Out of memory
Advertisements