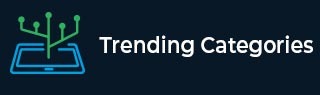
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can we change the id of an immutable string in Python?
In Python, a string is a sequence of characters. It's a type of data that represents text values, such as words, sentences, or even entire documents. Strings in Python are enclosed in either single quotes ('...') or double quotes ("..."), and can include alphanumeric characters, symbols, whitespace, and more.
As an immutable object, a string's ID cannot be changed in Python. As long as a new string that has the same value as the original string (valid only for small string literals), the ID of both the strings will remain same.
In Python, small string literals (strings with only ASCII characters) that have the same value are interned by the interpreter, meaning that they will have the same ID.
However, for larger strings or strings with non-ASCII characters, even if they have the same value, they will generally have different IDs because they will not be interned.
Here are three different ways to achieve this:
Using String Concatenation
Example
Here, we concatenate an empty string to the original string to create a new string with the same value. Since we are concatenating with an empty string, it doesn't change the value of the original string, so it creates a new string with the same ID.
# create a string my_string = "Hello, world!" # create a new string with the same value but a different ID using concatenation new_string = my_string + "" # check IDs of both strings print(my_string) print(new_string) print(id(my_string)) print(id(new_string))
Output
Hello, world! Hello, world! 140045518101040 140045518101040
Using string concatenation to create a new string
Example
In this example, we are creating a new string by concatenating the word "Goodbye" with a slice of the original string that starts at index 5 (i.e., after the "Hello" part). This creates a new string with the value "Goodbye, world!", which has a different ID than the original string.
# create a string my_string = "Hello, world!" # create a new string with a different ID new_string = "Goodbye" + my_string[5:] # print the original string and the new string print("Original string:", my_string) print("New string:", new_string) print(id(my_string)) print(id(new_string))
Output
Original string: Hello, world! New string: Goodbye, world! 139898304986544 139898304917744
Using the string replace() Method to Create a New String
Example
In this example, we are creating a new string by using the string replace() method to replace the word "Hello" in the original string with the word "Goodbye". This creates a new string with the value "Goodbye, world!", which has a different ID than the original string.
# create a string my_string = "Hello, world!" # create a new string with a different ID new_string = my_string.replace("Hello", "Goodbye") # print the original string and the new string print("Original string:", my_string) print("New string:", new_string) print(id(my_string)) print(id(new_string))
Output
Original string: Hello, world! New string: Goodbye, world! 139898304554928 139898304555120
Using string Formatting to Create a New String
Example
In this example, we are creating a new string by using string formatting to insert a slice of the original string into a new string template. The slice starts at index 7 (i.e., after the comma and space), so the new string has the value "Goodbye, world!". This new string has a different ID than the original string.
# create a string my_string = "Hello, world!" # create a new string with a different ID new_string = "Goodbye, {}".format(my_string[7:]) # print the original string and the new string print("Original string:", my_string) print("New string:", new_string) print(id(my_string)) print(id(new_string))
Output
Original string: Hello, world! New string: Goodbye, world! 139898304805808 139898304806256
Using the string() Constructor
Example
Here, we use the string constructor to create a new string with the same value. In Python, small string literals (strings with only ASCII characters) that have the same value are interned by the interpreter, meaning that they will have the same ID.
# create a string my_string = "Hello, world!" # create a new string with the same value using the string constructor new_string = str(my_string) # check IDs of both strings print(my_string) print(new_string) print(id(my_string)) print(id(new_string))
Output
Hello, world! Hello, world! 140704360063024 140704360063024
Using Slicing
Example
Here, we use slicing to create a new string with the same value as the original string. Slicing with no start or end index returns a copy of the original string.
In Python, small string literals (strings with only ASCII characters) that have the same value are interned by the interpreter, meaning that they will have the same ID.
# create a string my_string = "Hello, world!" # create a new string with the same value using slicing new_string = my_string[:] # check IDs of both strings print(my_string) print(new_string) print(id(my_string)) print(id(new_string))
Output
Hello, world! Hello, world! 140321283842096 140321283842096
Conclusion
In conclusion, if two string objects have the same value and that value is a small string literal, they will have the same ID. However, for larger strings or strings with non-ASCII characters, even if they have the same value, they will generally have different IDs because they will not be interned.