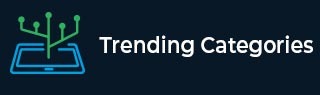
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can I fill out a Python string with spaces?
A string is a collection of characters that can represent a single word or a whole sentence. Unlike other technologies there is no need to be declared strings in Python explicitly we can define a string with or without the datatype specifier.
A string in Python is an object of the String class, which contains multiple methods using which you can manipulate and access strings.
In this article we are going to discuss fill out a Python string with spaces. Let’s see various solutions one by one.
Using the ljust(), rjust() and center() methods
To pad the string with desired characters python provides there methods namely, ljust(), rjust() and center().
The ljust() function is used to fill out the spaces to the right of the given string or pad them.
The rjust() function is used to fill out the spaces to the left of the given string or pad them.
The center() function is used to fill out the spaces to both left and right to the string or pad them otherwise.
All these have 2 parameters −
width − This represents the number of spaces you want to fill. This number is including the length of the string, if the number is less than the length of the string then there won’t be any change.
fillchar(optional) − This parameter represents a character we want to use as the padding. If nothing is specified, then the given string is padded with spaces.
Example 1
In the program given below, we are using the ljust() method for padding in the first case and we are filling with @ in the second case.
str1 = "Welcome to Tutorialspoint" str2 = str1.rjust(15) #str3 = str1.ljust(15,'@') print("Padding the string ",str1) print(str2) print("Filling the spaces of the string",str1) print(str1.rjust(15,'@'))
Output
The output of the above program is,
('Padding the string ', 'Welcome to Tutorialspoint') Welcome to Tutorialspoint ('Filling the spaces of the string', 'Welcome to Tutorialspoint') Welcome to Tutorialspoint
Example 2
In the program given below, we are using rjust() method for padding in the first case and we are filling with @ in the second case.
str1 = "Welcome to Tutorialspoint" str2 = str1.rjust(30) str3 = str1.rjust(30,'@') print("Padding the string ",str1) print(str2) print("Filling the spaces of the string",str1) print(str3)
Output
The output of the above program is,
('Padding the string ', 'Welcome to Tutorialspoint') Welcome to Tutorialspoint ('Filling the spaces of the string', 'Welcome to Tutorialspoint') @@@@@Welcome to Tutorialspoint
Example 3
In the program given below, we are using the center() method for padding in the first case and we are filling with @ in the second case.
str1 = "Welcome to Tutorialspoint" str2 = str1.center(30) str3 = str1.center(30,'@') print("Padding the string ",str1) print(str2) print("Filling the spaces of the string",str1) print(str3)
Output
The output of the above program is,
('Padding the string ', 'Welcome to Tutorialspoint') Welcome to Tutorialspoint ('Filling the spaces of the string', 'Welcome to Tutorialspoint') @@Welcome to Tutorialspoint@@@
Using the format() method
We can use the string format method for filling out spaces and padding the string. We perform the format() function mostly on a print statement.
We will mention the number of spaces to be filled in a curly brace using a colon for adding the right padding. To add left padding we should also add the > symbol and for centre padding, we should use the ^ operator.
For right padding use the following statement −
print('{:numofspaces}'.format(string))
For left padding use the following statement −
print('{:>numofspaces}'.format(string))
For centre padding use the following statement −
print('{:^numofspaces}'.format(string))
Example
In the example given below, we are using format method for right padding, left padding and centre padding.
str1 = "Welcome to Tutorialspoint" str2 = ('{:35}'.format(str1)) str3 = ('{:>35}'.format(str1)) str4 = ('{:^35}'.format(str1)) print("Right Padding of the string ",str1) print(str2) print("Left Padding of the string ",str1) print(str3) print("Center Padding of the string ",str1) print(str4)
Output
The output of the above program is,
('Right Padding of the string ', 'Welcome to Tutorialspoint') Welcome to Tutorialspoint ('Left Padding of the string ', 'Welcome to Tutorialspoint') Welcome to Tutorialspoint ('Center Padding of the string ', 'Welcome to Tutorialspoint') Welcome to Tutorialspoint