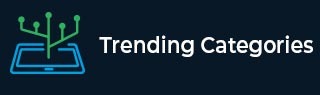
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can I convert the arguments object to an array in JavaScript?
This tutorial teaches us to convert the arguments object to array in JavaScript. Before we proceed with the tutorial, let’s look at the arguments object. In JavaScript, every function contains the ‘arguments’ object. It includes the values of all arguments we passed while calling or invoking the function.
It is very similar to an array, but it is not an actual array.
The ‘arguments’ object has a length property, and we can use it to know how many arguments are passed during the function call.
We can access all values of the ‘arguments’ object like an array index property. For example, arguments[0] give the first value of the object and so on.
We can use the Array methods such as slicer(), forEach(), map(), filter(), etc., on the arguments object.
So, to use all array methods to the arguments object, we need to convert it into the JavaScript array.
Here, we have different approaches to converting the arguments Object to JavaScript array.
Using the Array.from() Method
We will use the Array.from() method in this approach. It converts the iterable object or array-like objects to the array in JavaScript. It creates a copy of the objects and converts them into an array. We can access the converted array either directly or by storing it into another variable.
Syntax
Users can follow the below syntax to convert the arguments object to an array.
Array.from( arguments );
Parameters
arguments − arguments object to be converted to an array.
Return − Array.form() method returns an array from arguments object.
Example 1
The below example demonstrates the conversion of the arguments object to an array using the Array.from() method.
<html> <head> <title> Converting arguments Object to an array. </title> </head> <body> <h2>The Array.from() Method</h2> <p> The Array.from() method returns an array.</p> <p> Array created from arguments Object:</p> <p id="output"></p> <script > let outputDiv = document.getElementById("output"); function convertToArray() { // store the array of arguments object to resultantArray const resultantArray = Array.from(arguments); const mapArray = resultantArray.map((arg) => { return arg }); outputDiv.innerHTML = mapArray; } // call function by passing arguments convertToArray(10,20,30); </script> </body> </html>
In the above code, users can see that we have passed 10, 20, and 30 as an argument of the function. In the function, we have converted the arguments object to an array and use the map function with the converted array to ensure that it is appropriately converted.
Using the rest parameters
The rest parameter is the syntax in the JavaScript introduced in the ES6. We can specify the unknown number of arguments in the array form using the rest parameter. Users just need to use the spread operator (...) with the last parameters.
In our case, we need to specify the spread operator with the first parameter to get the array of all arguments.
Syntax
Users can follow the below syntax.
function demo( ...args ) { // function body } demo(1,3,5);
Parameters
args − Users can use any variable name instead of args, But to access the arguments in the array, users need to use the same array name inside the function.
Return − It will return an array. When we pass rest parameters to a function, it converts the parameters object to an array automatically.
Example 2
The below example demonstrate the use of the spread operator to convert the arguments object to array.
<html> <head> <title> Converting arguments Object to an array. </title> </head> <body> <h2>Rest Arguments: Converting arguments Object to an array.</h2> <p> A fucntion with rest paramters treats the arguments object as an array.</p> <p> Array created from arguments Object: </p> <p id="output"></p> <script> function convertToArray(...args) { document.getElementById("output").innerHTML = args; } // call function by passing arguments convertToArray("tutorials", "point", "simply", "easy", "learning"); </script> </body> </html>
Users can see that we have converted the arguments object into an array using the spread operator (…).
By creating a new array
In this method, we will create a new array and assigns the values of the arguments’ object to the new array. As users know, we can access the values of arguments object by indexing which will help us add values to the new array.
Syntax
Users can follow the below syntax.
newArray[i] = arguments[i]
Algorithm
- Step 1 − Create a new empty array.
- Step 2 − Iterate through the object and access values of the object one by one and add them to the array.
- Step 3 − We have converted the arguments’ object to array and users can use all array methods with the newly created array.
Example 3
The below example demonstrates converting the arguments object to an array without using the built-in method in JavaScript.
<html> <body> <h2>Converting arguments Object to an array.</h2> <p> Newly created array after applying the sort() method: </p> <p id="result"> </p> <script> let resultDiv = document.getElementById("result"); function convertToArray() { // assign arguments values to the newArray const newArray = []; for (var i = 0; i < arguments.length; i++) { newArray[i] = arguments[i]; } // sort the newArray const sortedArray = newArray.sort((a, b) => a - b); resultDiv.innerHTML = sortedArray; } // call function by passing arguments convertToArray(1, 354, 23, 132, 64, 2, 7, 3, 8, 10); </script> </body> </html>
Users can take a look that we have converted arguments object to array manually and sort the newly created array, which prints the above output.
Conclusion
In this tutorial, we have gone through the three methods to convert the arguments’ object to the array. The first two approaches use the built-in method to convert objects to the array. In the third approach, we manually converted the arguments object to an array. The best approach is for users can use the rest parameter to perform the above operation.