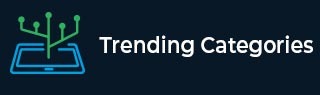
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Can we define a class inside a Java interface?
Yes, you can define a class inside an interface. In general, if the methods of the interface use this class and if we are not using it anywhere else we will declare a class within an interface.
Example
interface Library { void issueBook(Book b); void retrieveBook(Book b); public class Book { int bookId; String bookName; int issueDate; int returnDate; } } public class Sample implements Library { public void issueBook(Book b) { System.out.println("Book Issued"); } public void retrieveBook(Book b) { System.out.println("Book Retrieved"); } public static void main(String args[]) { Sample obj = new Sample(); obj.issueBook(new Library.Book()); obj.retrieveBook(new Library.Book()); } }
Output
Hello welcome to tutorialspoint
If we need to provide a default implementation of the interface, we will define a class inside an interface as:
Example
interface Library { void issueBook(Book b); void retrieveBook(Book b); public class Book implements Library { int bookId; String bookName; int issueDate; int returnDate; public void issueBook(Book b) { System.out.println("book issued"); } public void retrieveBook(Book b) { System.out.println("book retrieved"); } } } public class Sample { public void demo() { System.out.println("Hello welcome to tutorialspoint"); } public static void main(String args[]) { Sample obj = new Sample(); obj.demo(); } }
Advertisements