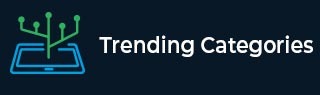
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
8085 Program to Add N numbers, of size 8 bits
In this program, we will see how to add a block of data using the 8085 microprocessor.
Problem Statement
Write 8085 Assembly language program to add N 1-byte numbers. The value of N is provided.
Discussion
In this problem, we are using location 8000H to hold the length of the block. The main block is stored from address 8010H. We are storing the result at location 9000H and 9001H. The 9000H holding the lower byte, and 9001H is holding the upper byte.
Repeatedly we are taking the number from the memory, then adding it with the accumulator and increase the register E content when carry flag is set. Initially, Eis cleared.
Input
Address | Data |
---|---|
. . . | . . . |
8000 | 08 |
. . . | . . . |
8010 | AF |
8011 | 2E |
8012 | 7C |
8013 | 81 |
8014 | 2C |
8015 | BF |
8016 | FB |
8017 | 1C |
. . . | . . . |
Flow Diagram
Program
Address | HEX Codes | Labels | Mnemonics | Comments | |
---|---|---|---|---|---|
F000 | 21, 00, 80 | LXI H, 8000H | Load the address to get a count of numbers | ||
F003 | 4E | MOV C, M | Load C with the count value | ||
F004 | 21,10, 80 | LXI H, 8010H | Load HL with the starting address | ||
F007 | AF | XRA A | Clear Accumulator | ||
F008 | 5F | MOV E, A | Clear the E register also | ||
F009 | 86 | LOOP | ADD M | Add Memory content with Accumulator | |
F00A | D2, 0E, F0 | JNC SKIP | When the carry is false, skip next task | ||
F00D | 1C | INR E | Increase E, when the C flag is set | ||
F00E | 0D | SKIP | DCR C | Decrease C register by 1 | |
F00F | 23 | INX H | Point to next location | ||
F010 | C2, 09, F0 | JNZ LOOP | When Zero is false, go to LOOP | ||
F013 | 21, 00, 90 | LXIH, 9000H | Load address to store the result | ||
F016 | 77 | MOV M, A | Save accumulator content | ||
F017 | 23 | INX H | Increase HL pair | ||
F018 | 73 | MOV M, E | Store carry | ||
F019 | 76 | HLT | Terminate the program |
Output
Address | Data |
---|---|
. . . | . . . |
9000 | DC |
9001 | 03 |
. . . | . . . |
Advertisements