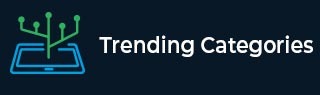
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
8085 code to convert binary number to ASCII code
Now let us see a program of Intel 8085 Microprocessor. This program will convert binary or hexadecimal number to ASCII values.
Problem Statement
Write 8085 Assembly language program to convert binary or Hexadecimal characters to ASCII values.
Discussion
We know that the ASCII of number 00H is 30H (48D), and ASCII of 09H is39H (57D). So all other numbers are in the range 30H to 39H. TheASCII value of 0AH is 41H (65D) and ASCII of 0FH is 46H (70D), so all other alphabets (B, C, D, E, F) are in the range 41H to 46H.
Here we are providing hexadecimal digit at memory location 8000H, The ASCII equivalent is storing at location 8001H.
The logic behind HEX to ASCII conversion is very simple. We are just checking whether the number is in range 0 – 9 or not. When the number is in that range, then the hexadecimal digit is numeric, and we are just simply adding 30H with it to get the ASCII value. When the number is not in range 0 – 9, then the number is range A – F,so for that case, we are converting the number to 41H on wards.
In the program at first we are clearing the carry flag. Then subtracting 0AHfrom the given number. If the value is numeric, then after subtraction the result will be negative, so the carry flag will beset. Now by checking the carry status we can just add 30H with the value to get ASCII value.
In other hand when the result of subtraction is positive or 0, then we are adding 41H with the result of the subtraction.
Input
first input
Address | Data |
---|---|
. . . | . . . |
8000 | 0A |
. . . | . . . |
second input
Address | Data |
---|---|
. . . | . . . |
8000 | 05 |
. . . | . . . |
third input
Address | Data |
---|---|
. . . | . . . |
8000 | 0F |
. . . | . . . |
Flow Diagram
Program
Address | HEX Codes | Labels | Mnemonics | Comments |
---|---|---|---|---|
F000 | 21, 00, 80 | LXI H, 8000H | Load address of the number | |
F003 | 7E | MOV A,M | Load Acc with the data from memory | |
F004 | 47 | MOV B,A | Copy the number into B | |
F005 | 37 | STC | Set CarryFlag | |
F006 | 3F | CMC | ComplementCarry Flag | |
F007 | D6, 0A | SUI 0AH | Subtract 0AHfrom A | |
F009 | DA, 11, F0 | JC NUM | When carry is present, A is numeric | |
F00C | C6, 41 | ADI 41H | Add 41H forAlphabet | |
F00E | C3, 14, F0 | JMP STORE | Jump to store the value | |
F011 | 78 | NUM | MOV A, B | Get back B toA |
F012 | C6 | ADI 30H | Add 30H withA to get ASCII | |
F014 | 23 | STORE | INX H | Point to next location to store address |
F015 | 77 | MOV M,A | Store A to memory location pointed by HL pair | |
F016 | 76 | HLT | Terminate the program |
Output
first output
Address | Data |
---|---|
. . . | . . . |
8001 | 41 |
. . . | . . . |
second output
Address | Data |
---|---|
. . . | . . . |
8001 | 35 |
. . . | . . . |
third output
Address | Data |
---|---|
. . . | . . . |
8001 | 46 |
. . . | . . . |