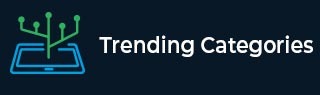
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
01 Matrix in C++
Suppose we have a matrix consists of 0 and 1, we have to find the distance of the nearest 0 for each cell. Here the distance between two adjacent cells is 1.
So, if the input is like
0 | 0 | 0 |
0 | 1 | 0 |
1 | 1 | 1 |
then the output will be
0 | 0 | 0 |
0 | 1 | 0 |
1 | 2 | 1 |
To solve this, we will follow these steps −
Define an array dir of size: 4 x 2 := {{1, 0}, { - 1, 0}, {0, - 1}, {0, 1}}
n := row count, m := column count
Define one matrix ret of order (n x m) and fill this with inf
Define one queue q
for initialize i := 0, when i < n, update (increase i by 1), do −
for initialize j := 0, when j < m, update (increase j by 1), do −
if not matrix[i, j] is non-zero, then −
ret[i, j] := 0
insert {i, j} into q
for initialize lvl := 1, when not q is empty, update (increase lvl by 1), do −
sz := size of q
while sz is non-zero, decrease sz by 1 in each iteration, do −
Define one pair curr := front element of q
delete element from q
for initialize k := 0, when k < 4, update (increase k by 1), do −
nx := curr.first + dir[k, 0]
ny := curr.second + dir[k, 1]
if nx < 0 or nx >= n or ny < 0 or ny >= m or ret[nx, ny] < lvl, then −
ret[nx, ny] := lvl
insert {nx, ny} into q
return ret
Example
Let us see the following implementation to get better understanding −
#include <bits/stdc++.h> using namespace std; void print_vector(vector<vector<auto> > v){ cout << "["; for(int i = 0; i<v.size(); i++){ cout << "["; for(int j = 0; j <v[i].size(); j++){ cout << v[i][j] << ", "; } cout << "],"; } cout << "]"<<endl; } int dir[4][2] = {{1, 0}, {-1, 0}, {0, -1}, {0, 1}}; class Solution { public: vector<vector<int>> updateMatrix(vector<vector<int>>& matrix) { int n = matrix.size(); int m = matrix[0].size(); vector < vector <int> > ret(n, vector <int>(m, INT_MAX)); queue < pair <int, int> > q; for(int i = 0; i < n; i++){ for(int j = 0; j < m; j++){ if(!matrix[i][j]){ ret[i][j] = 0; q.push({i, j}); } } } for(int lvl = 1; !q.empty(); lvl++){ int sz = q.size(); while(sz--){ pair <int, int> curr = q.front(); q.pop(); for(int k = 0; k < 4; k++){ int nx = curr.first + dir[k][0]; int ny = curr.second + dir[k][1]; if(nx < 0 || nx >= n || ny < 0 || ny >= m || ret[nx][ny] < lvl) continue; ret[nx][ny] = lvl; q.push({nx, ny}); } } } return ret; } }; main(){ Solution ob; vector<vector<int>> v = {{0,0,0},{0,1,0},{1,1,1}}; print_vector(ob.updateMatrix(v)); }
Input
{{0,0,0},{0,1,0},{1,1,1}}
Output
[[0, 0, 0, ],[0, 1, 0, ],[1, 2, 1, ],]