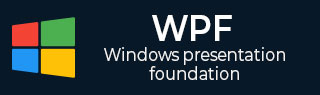
- WPF - Home
- WPF - Overview
- WPF - Environment Setup
- WPF - Hello World
- WPF - XAML Overview
- WPF - Elements Tree
- WPF - Dependency Properties
- WPF - Routed Events
- WPF - Controls
- WPF - Layouts
- WPF - Nesting Of Layout
- WPF - Input
- WPF - Command Line
- WPF - Data Binding
- WPF - Resources
- WPF - Templates
- WPF - Styles
- WPF - Triggers
- WPF - Debugging
- WPF - Custom Controls
- WPF - Exception Handling
- WPF - Localization
- WPF - Interaction
- WPF - 2D Graphics
- WPF - 3D Graphics
- WPF - Multimedia
WPF - Keyboard
There are many types of keyboard inputs such as KeyDown, KeyUp, TextInput, etc. In the following example, some of the keyboard inputs are handled. The following example defines a handler for the Click event and a handler for the KeyDown event.
Lets create a new WPF project with the name WPFKeyboardInput.
Drag a textbox and a button to a stack panel and set the following properties and events as shown in the following XAML file.
<Window x:Class = "WPFKeyboardInput.MainWindow" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local = "clr-namespace:WPFKeyboardInput" mc:Ignorable = "d" Title = "MainWindow" Height = "350" Width = "604"> <Grid> <StackPanel Orientation = "Horizontal" KeyDown = "OnTextInputKeyDown"> <TextBox Width = "400" Height = "30" Margin = "10"/> <Button Click = "OnTextInputButtonClick" Content = "Open" Margin = "10" Width = "50" Height = "30"/> </StackPanel> </Grid> </Window>
Here is the C# code in which different keyboard and click events are handled.
using System.Windows; using System.Windows.Input; namespace WPFKeyboardInput { /// <summary> /// Interaction logic for MainWindow.xaml /// </summary> public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } private void OnTextInputKeyDown(object sender, KeyEventArgs e) { if (e.Key == Key.O && Keyboard.Modifiers == ModifierKeys.Control) { handle(); e.Handled = true; } } private void OnTextInputButtonClick(object sender, RoutedEventArgs e) { handle(); e.Handled = true; } public void handle() { MessageBox.Show("Do you want to open a file?"); } } }
When the above code is compiled and executed, it will produce the following window −

If you click the Open button or press CTRL+O keys inside textbox, it will display the same message.

wpf_input.htm
Advertisements