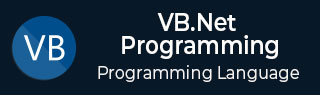
- VB.Net Basic Tutorial
- VB.Net - Home
- VB.Net - Overview
- VB.Net - Environment Setup
- VB.Net - Program Structure
- VB.Net - Basic Syntax
- VB.Net - Data Types
- VB.Net - Variables
- VB.Net - Constants
- VB.Net - Modifiers
- VB.Net - Statements
- VB.Net - Directives
- VB.Net - Operators
- VB.Net - Decision Making
- VB.Net - Loops
- VB.Net - Strings
- VB.Net - Date & Time
- VB.Net - Arrays
- VB.Net - Collections
- VB.Net - Functions
- VB.Net - Subs
- VB.Net - Classes & Objects
- VB.Net - Exception Handling
- VB.Net - File Handling
- VB.Net - Basic Controls
- VB.Net - Dialog Boxes
- VB.Net - Advanced Forms
- VB.Net - Event Handling
- VB.Net Advanced Tutorial
- VB.Net - Regular Expressions
- VB.Net - Database Access
- VB.Net - Excel Sheet
- VB.Net - Send Email
- VB.Net - XML Processing
- VB.Net - Web Programming
- VB.Net Useful Resources
- VB.Net - Quick Guide
- VB.Net - Useful Resources
- VB.Net - Discussion
VB.Net - Arithmetic Operators
Following table shows all the arithmetic operators supported by VB.Net. Assume variable A holds 2 and variable B holds 7, then −
Operator | Description | Example |
---|---|---|
^ | Raises one operand to the power of another | B^A will give 49 |
+ | Adds two operands | A + B will give 9 |
- | Subtracts second operand from the first | A - B will give -5 |
* | Multiplies both operands | A * B will give 14 |
/ | Divides one operand by another and returns a floating point result | B / A will give 3.5 |
\ | Divides one operand by another and returns an integer result | B \ A will give 3 |
MOD | Modulus Operator and remainder of after an integer division | B MOD A will give 1 |
Example
Try the following example to understand all the arithmetic operators available in VB.Net −
Module operators Sub Main() Dim a As Integer = 21 Dim b As Integer = 10 Dim p As Integer = 2 Dim c As Integer Dim d As Single c = a + b Console.WriteLine("Line 1 - Value of c is {0}", c) c = a - b Console.WriteLine("Line 2 - Value of c is {0}", c) c = a * b Console.WriteLine("Line 3 - Value of c is {0}", c) d = a / b Console.WriteLine("Line 4 - Value of d is {0}", d) c = a \ b Console.WriteLine("Line 5 - Value of c is {0}", c) c = a Mod b Console.WriteLine("Line 6 - Value of c is {0}", c) c = b ^ p Console.WriteLine("Line 7 - Value of c is {0}", c) Console.ReadLine() End Sub End Module
When the above code is compiled and executed, it produces the following result −
Line 1 - Value of c is 31 Line 2 - Value of c is 11 Line 3 - Value of c is 210 Line 4 - Value of d is 2.1 Line 5 - Value of c is 2 Line 6 - Value of c is 1 Line 7 - Value of c is 100
vb.net_operators.htm
Advertisements