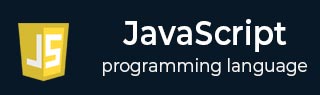
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript String substring() Method
The JavaScript String substring() method is used to retrieve a part of a string from the original string starting from the specified startIndex up to indexEnd(excluding this), and if the indexEnd is not specified, it extracted to the end of the string.
If the indexStart value is greater than the indexEnd parameter value, then the arguments will be swapped. For example, if indexStart = 5, and indexEnd = 2, then (5, 2) is equivalent to (2, 5). If either indexStart or indexEnd is less than zero, it is treated as 0.
Syntax
Following is the syntax of JavaScript String substring() method −
substring(indexStart, indexEnd)
Parameters
This method accepts two parameters: 'indexStart' and 'indexEnd', which are described below −
- indexStart − The starting position from where string start extracted.
- indexEnd − The end position from where up to the string will be extracted excluding this.
Return value
This method returns a new string from the original string between two indices.
Example 1
If we omit the indexEnd parameter, then the sustring() method extracts a substring starting from the specified indexStart position to the end of the string.
In the following program, we are using the JavaScript String substring() method to extract a part of the string from the original string "Tutorials Point". The extraction starts from the specified indexStart 10 and continues till the end of the string.
<html> <head> <title>JavaScript String substring() Method</title> </head> <body> <script> const str = "Tutorials Point"; let indexStart = 10; document.write("Original string: ", str); document.write("<br>Start index: ", indexStart); document.write("<br>Extracted string: ", str.substring(indexStart)); </script> </body> </html>
Output
The above program returns a new string as "Point".
Original string: Tutorials Point Start index: 10 Extracted string: Point
Example 2
If we pass both indexStart and indexEnd parameters to this method, it extracts the string starting from the indexStart and up to indexEnd(excluding this).
Here is another example of the JavaScript substring() method. We use this method to extract a part of the string from the string "Hypertext Markup Language" between the indices 5 and 15.
<html> <head> <title>JavaScript String substring() Method</title> </head> <body> <script> const str = "Hypertext Markup Language"; let indexStart = 5; let indexEnd = 16; document.write("Original string: ", str); document.write("<br>Indices are: ", indexStart, ", ", indexEnd); document.write("<br>Extracted string: ", str.substring(indexStart, indexEnd)); </script> </body> </html>
Output
After executing the above program, it returns a new string as −
Original string: Hypertext Markup Language Indices are: 5, 16 Extracted string: text Markup
Example 3
If the indexStart value is greater than the indexEnd parameter value, then the argument will be swapped.
As discussed, if indexStart is greater than indexEnd, then the arguments will be swapped so that the indices (5, 2) will be swapped and equal to the indices (2, 5).
<html> <head> <title>JavaScript String substring() Method</title> </head> <body> <script> const str = "Hello World"; let indexStart = 5; let indexEnd = 2; document.write("Original string: ", str); document.write("<br>Indices are: ", indexStart, ", ", indexEnd); document.write("<br>Extracted string(2, 5): ", str.substring(indexStart, indexEnd)); </script> </body> </html>
Output
The above program returns a new substring "llo".
Original string: Hello World Indices are: 5, 2 Extracted string(2, 5): llo