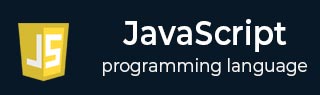
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript String replace() Method
The JavaScript String replace() method searches for a value or a regular expression and returns a new string by replacing the first occurrence of a pattern with a specified replacement. The pattern can be either a string or a regular expression, and the replacement can be either a string or a function. It does not change the original string value, but returns a new string.
If the pattern is string, then only the first occurance will be removed.
Following is the difference between replace() and replaceAll() method −
The replaceAll() method replaces all occurrences of a search value or a regex with a specified replacement, for example: "_tutorials_point_".replaceAll("_", "") returns "tutorialspoint", whereas the replace() method replaces only the first occurrence of a search value or a regex with a specified replacement.
Syntax
Following is the syntax of JavaScript String replace() method −
replace(pattern, replacement)
Parameters
This method accepts two parameters such as: 'pattern' and 'replacement', which are described below −
- pattern − The string or a regular expression replaced by the replament.
- replacement − The string or function to be replaced the pattern.
Return value
This method returns a new string with the first occurrence of a pattern replaced by a specified value.
Example 1
In this program, we are using the JavaScript String replace() method to replace a string "Point" with "point" in the string "Tutorials Point".
<html> <head> <title>JavaScript String replace() Method</title> </head> <body> <script> const str = "Tutorials Point"; document.write("Original string: ", str); document.write("<br>New string after replacement: ", str.replace("Point", "point")); </script> </body> </html>
Output
The above program returns "Tutorials point" after replacement −
Original string: Tutorials Point New string after replacement: Tutorials point
Example 2
Here is another example of the JavaScript String replace() method. In this example, we use the replace() method to replace the first occurrence of whitespace (" ") with an empty string ("") in the string " Hello World ".
<html> <head> <title>JavaScript String replace() Method</title> </head> <body> <script> const str = " Hello World "; document.write("Original string: ", str); document.write("<br>New string after replacement: ", str.replace(" ", "")); </script> </body> </html>
Output
After executing the above program, it returns a new string "Hello World " after replace.
Original string: Hello World New string after replacement: Hello World
Example 3
When the search (pattern) value is an empty string, the replace() method inserts the replacement value at the beginning of the string, before any other characters.
<html> <head> <title>JavaScript String replace() Method</title> </head> <body> <script> const str = "TutorialsPoint"; document.write("Original string: ", str); document.write("<br>New string after replace: ", str.replace("", "__")); </script> </body> </html>
Output
Once the above program is executed, it returns " __TutorialsPoint".
Original string: TutorialsPoint New string after replace: __TutorialsPoint
Example 4
In the following program, we specify a string "$2" as the replacement argument to the replace() method. The regular expression does not have a second group, so it replaces and returns "$2oo".
<html> <head> <title>JavaScript String replace() Method</title> </head> <body> <script> const str = "foo"; let replacement = "$2"; document.write("Original string: ", str); document.write("<br>Replacement: ", replacement); document.write("<br>New String after replacement: ", str.replace(/(f)/, replacement)); </script> </body> </html>
Output
The above program returns "$2oo".
Original string: foo Replacement: $2 New String after replacement: $2oo