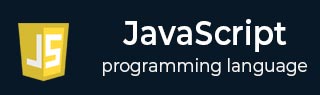
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript String localeCompare() Method
The JavaScript String localeCompare() method is used to compare two strings in the current locales that your browser is currently using. It returns a number that indicates whether this string comes before, after, or is the same as the given string in sort order.
The following is a list of statements that describe the number returned by this method −
- A positive number (+1) indicates that the given string occurs after the compare string.
- A negative number (-1), indicates that the given string occurs before the compare string.
- A zero (0) will be returned if both strings are equivalent to each other.
Syntax
Following is the syntax of JavaScript String localeCompare() method −
localeCompare(compareString, locales, options)
Parameters
This method accepts three parameters named 'compareString', 'locales', and 'options', which are described below −
- compareString − The string needs to be compared.
- locales (optional) − The locales of the current browser.
- options (optional) − An object adjusting the output format.
Return value
This method returns a number 0 if both strings are equivalent, -1 if the given string occurs before the compare string, or +1 if the given string occurs after the compare string.
Example 1
When no locales or options are specified, it performs a basic comparison based on the Unicode values of the characters in the strings. If the strings are equivalent, it returns 0; otherwise, it returns a positive or negative value indicating their order.
In the following program, we are using the JavaScript localeCompare() method to compare the string "Tutorialspoint" with the given string "Tutorialspoint".
<html> <head> <title>JavaScript String localeCompare() Method</title> </head> <body> <script> const str = "TutorialsPoint"; const compare_str = "TutorialsPoint"; document.write("Original string: ", str); document.write("<br>Compare string: ", compare_str); document.write("<br>The str.localeCompare(compare_str) method returns: ", str.localeCompare(compare_str)); </script> </body> </html>
Output
The above programs returns 0.
Original string: TutorialsPoint Compare string: TutorialsPoint The str.localeCompare(compare_str) method returns: 0
Example 2
If the given string occurs after the compare string, the localeCompare() method returns 1.
The following is another example of the JavaScript String localeCompare() method. In this example, we use this method to compare the string "hello" with the given string "HELLO" using the current locales "en".
<html> <head> <title>JavaScript String localeCompare() Method</title> </head> <body> <script> const str = "HELLO"; const compare_str = "hello"; const locale = "en"; document.write("Original string: ", str) document.write("<br>Compare string: ", compare_str); document.write("<br>Current locales: ", locale); document.write("<br>The str.localeCompare(compare_str) method returns: ", str.localeCompare(compare_str, locale)); </script> </body> </html>
Output
After executing the above program, it will return 1.
Original string: HELLO Compare string: hello Current locales: en The str.localeCompare(compare_str) method returns: 1
Example 3
When we pass all three parameters compareString, locales, and options to the localeCompare() method, it compares the compareString with the given string using the current locales, and the output format will be adjusted based on the specified options.
<html> <head> <title>JavaScript String localeCompare() Method</title> </head> <body> <script> const str = "tutorialspoint"; const compare_str = "TUTORIALSPOINT"; const locale = "en"; const option = {sensitivity: 'base'}; document.write("Original string: ", str) document.write("<br>Compare string: ", compare_str); document.write("<br>Current locales: ", locale); document.write("<br>Options: ", option.sensitivity); document.write("<br>The str.localeCompare(compare_str) method returns: ", str.localeCompare(compare_str)); document.write("<br>The str.localeCompare(compare_str, locale, option) method returns: ", str.localeCompare(compare_str, locale, option)); </script> </body> </html>
Output
The above program produces the following output −
Original string: tutorialspoint Compare string: TUTORIALSPOINT Current locales: en Options: base The str.localeCompare(compare_str) method returns: -1 The str.localeCompare(compare_str, locale, option) method returns: 0