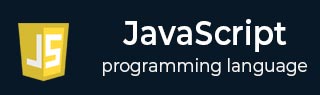
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript String length Property
The JavaScript String length (or string.length) property is used to find the number of characters present in the current string.
In JavaScript, a string is a data-type representing a sequence of characters. Strings can consist of letters, numbers, symbols, words, or sentences.
Syntax
Following is the syntax of JavaScript String length property −
string.length
Here, the string refers to the given string whose length you want to find.
Parameters
- It does not accept any parameter.
Return value
This property returns the length of the string, i.e., the number of characters present in it.
Example 1
If the given string is empty, it will return zero (0) as string length.
In the following program, we are using the JavaScript String length property to find the length of an empty string ("").
<html> <head> <title>JavaScript String length Property</title> </head> <body> <script> const str = ""; document.write("The given string: ", str); document.write("<br>Length of the given string: ", str.length); </script> </body> </html>
Output
The above program returns the string length as 0.
The given string: Length of the given string: 0
Example 2
The following is another example of the JavaScript String length property. We use the string.length property to find the number of characters present in the current string 'Tutorials point'.
The given string has 14 characters in it, but it will return the string length as 15 because it considers the white-space between the words as a character.
<html> <head> <title>JavaScript String length Property</title> </head> <body> <script> const str = "Tutorials point"; document.write("The given string: ", str); document.write("<br>Length of the given string: ", str.length); </script> </body> </html>
Output
After executing the above program, it will return the length of the string as −
The given string: Tutorials point Length of the given string: 15
Example 3
If the given string is empty but contains white spaces, it will consider all white spaces as characters and return the length of the string.
In the example below, we use the string.length property to retrieve the length of the string " " (which contains only white spaces).
<html> <head> <title>JavaScript String length Property</title> </head> <body> <script> const str = " "; document.write("The given string: ", str); document.write("<br>Length of the given string: ", str.length); </script> </body> </html>
Output
Once the above program is executed, it will return the string length.
The given string: Length of the given string: 4
Example 4
Let's compare the string length using the string.length property and use the result in a conditional statement to check whether the strings have equal length or not.
<html> <head> <title>JavaScript String length Property</title> </head> <body> <script> const str1 = "Tutorials Point"; const str2 = "Hello"; const str3 = "World"; document.write("The given strings are : ", str1, ", ", str2, ", ", str3); const len1 = str1.length; const len2 = str2.length; const len3 = str3.length; document.write("<br>Length of ", str1, " is: ", len1); document.write("<br>Length of ", str2, " is: ", len2); document.write("<br>Length of ", str3, " is: ", len3); if(len1 == len2){ document.write("<br>String ", str1, " and ", str2, " having equal length"); } else if(len1 == len3){ document.write("<br>String ", str1, " and ", str3, " having equal length"); } else if(len2 == len3){ document.write("<br>String ", str2, " and ", str3, " having equal length"); } else{ document.write("<br>None of the strings having equal length"); } </script> </body> </html>
Output
Following is the output of the above program −
The given strings are : Tutorials Point, Hello, World Length of Tutorials Point is: 15 Length of Hello is: 5 Length of World is: 5 String Hello and World having equal length