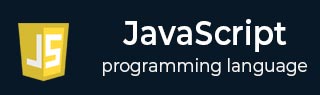
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript String lastIndexOf() Method
The JavaScript String lastIndexOf() method is used to find the index of the last occurrence of the specified substring in the original string. It returns the position of the substring, or -1 if the substring is not present. For example, "hello".lastIndexOf("ll") returns 2, while "hello".lastIndexOf("hi") returns -1.
This method accepts an optional parameter named position, which specifies the position in the original string from where the method starts searching for the specified substring. The default value of this parameter is 0. For example, "hello".lastIndexOf("l", 2) returns 2, while "hello".lastIndexOf("l", 3) returns 3.
Note − This method is case sensitive, it treats "a" and "A" as different values.
Syntax
Following is the syntax of JavaScript String lastIndexOf() method −
lastIndexOf(searchString, position)
Parameters
- searchString − The substring to be searched.
- position (optional) − The position to start from searching.
Return value
This method returns an index of the last occurrence of substring found.
Example 1
If we omit the position parameter, this method considers the default value 0 for position and returns the index of the last occurrence of the specified substring "t" in the original string "TutorialsPoint".
<html> <head> <title>JavaScript String lastIndexOf() Method</title> </head> <body> <script> const str = "TutorialsPoint"; const sub_str = "t"; document.write("Original string: ", str); document.write("<br>Sub-string to be searched: ", sub_str); document.write("<br>An index of search string '", sub_str,"' is: ", str.lastIndexOf(sub_str)); </script> </body> </html>
Output
The above program returns an index as 13 for substing "t".
Original string: TutorialsPoint Sub-string to be searched: t An index of search string 't' is: 13
Example 2
If we pass the position parameter value as 5, the method will start searching for the substring from the position 5 in the original string.
Following is another example of the JavaScript String lastIndexOf() method. Here, we are using this method to find the index of the substring "o" at the starting position 5 in the original string "HelloWorld".
<html> <head> <title>JavaScript String lastIndexOf() Method</title> </head> <body> <script> const str = "HelloWorld"; const sub_str = "o"; const position = 5; document.write("Original string: ", str); document.write("<br>Sub-string to be searched: ", sub_str); document.write("<br>Position value is: ", position); document.write("<br>An index of search string '", sub_str,"' is: ", str.lastIndexOf(sub_str, position)); </script> </body> </html>
Output
After executing the above program, it returns an index value of '4' for the substring 'o' at the specified position 5.
Original string: HelloWorld Sub-string to be searched: o Position value is: 5 An index of search string 'o' is: 4
Example 3
If the specified substring is not found in the original string, this method returns -1.
In the Following example, we are trying to find an index of sub-string "Mom", in the original string "JavaScript".
<html> <head> <title>JavaScript String lastIndexOf() Method</title> </head> <body> <script> const str = "JavaScript"; const sub_str = "Mom"; document.write("Original string: ", str); document.write("<br>Sub-string to be searched: ", sub_str); let result = str.lastIndexOf(sub_str); document.write("<br>Method str.lastIndexOf(sub_str) returns: ", result); if(result == -1){ document.write("<br>Not found...!"); } else{ document.write("<br>Found...!"); } </script> </body> </html>
Output
The lastIndexOf() method in the above program returns -1, when the substring is not found.
Original string: JavaScript Sub-string to be searched: Mom Method str.lastIndexOf(sub_str) returns: -1 Not found...!
Example 4
Let's see the difference between the indexOf() and lastIndexOf() methods by executing the following program.
<html> <head> <title>JavaScript String lastIndexOf() Method</title> </head> <body> <script> const str = "JavaScript"; const sub_str = "a"; const position = 20; document.write("Original string: ", str); document.write("<br>Sub-string to be searched: ", sub_str); document.write("<br>Position value: ", position); let result = str.lastIndexOf(sub_str, position); document.write("<br>Method str.lastIndexOf(sub_str) returns: ", result); </script> </body> </html>
Output
The indexOf() and lastIndexOf() methods return different index values for the same sub-string as −
Original string: JavaScript Sub-string to be searched: a Position value: 20 Method str.lastIndexOf(sub_str) returns: 3