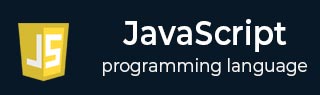
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Roadmap
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Safe Assignment Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Asynchronous
- JavaScript - Asynchronous
- JavaScript - Callback Functions
- JavaScript - Promises
- JavaScript - Async/Await
- JavaScript - Microtasks
- JavaScript - Promisification
- JavaScript - Promises Chaining
- JavaScript - Timing Events
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods & Properties
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Attributes (Attr)
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript Advanced Chapters
- JavaScript - Bubble Sort Algorithm
- JavaScript - Circular Reference Error
- JavaScript - Code Testing with Jest
- JavaScript - CORS Handling
- JavaScript - Data Analysis
- JavaScript - Dead Zone
- JavaScript - Design Patterns
- JavaScript - Engine and Runtime
- JavaScript - Execution Context
- JavaScript - Function Composition
- JavaScript - Immutability
- JavaScript - Kaboom.js
- JavaScript - Lexical Scope
- JavaScript - Local Storage
- JavaScript - Memoization
- JavaScript - Minifying JS
- JavaScript - Mutability vs Immutability
- JavaScript - Package Manager
- JavaScript - Parse S-Expressions
- JavaScript - Prototypal Inheritance
- JavaScript - Reactivity
- JavaScript - Require Function
- JavaScript - Selection API
- JavaScript - Session Storage
- JavaScript - SQL CRUD Operations
- JavaScript - Supercharged Sorts
- JavaScript - Temporal Dead Zone
- JavaScript - Throttling
- JavaScript - TRPC Library
- JavaScript - Truthy and Falsy Values
- JavaScript - Upload Files
- JavaScript - Date Comparison
- JavaScript - Recursion
- JavaScript - Data Structures
- JavaScript - Base64 Encoding
- JavaScript - Callback Function
- JavaScript - Current Date/Time
- JavaScript - Date Validation
- JavaScript - Filter Method
- JavaScript - Generating Colors
- JavaScript - HTTP Requests
- JavaScript - Insertion Sort
- JavaScript - Lazy Loading
- JavaScript - Linked List
- JavaScript - Nested Loop
- JavaScript - Null Checking
- JavaScript - Get Current URL
- JavaScript - Graph Algorithms
- JavaScript - Higher Order Functions
- JavaScript - Empty String Check
- JavaScript - Form Handling
- JavaScript - Functional Programming
- JavaScript - Parameters vs Arguments
- JavaScript - Prototype
- JavaScript - Reactive Programming
- JavaScript - Reduce Method
- JavaScript - Rest Operator
- JavaScript - Short Circuiting
- JavaScript - Undefined Check
- JavaScript - Unit Testing
- JavaScript - Validate URL
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
JavaScript Math.random() Method
The Math.random() method in JavaScript is used to generate a pseudo-random decimal number between 0 (inclusive) and 1 (exclusive).
Note: The Math.random() is not suitable for cryptographic or security-related purposes. The numbers generated by Math.random() are not truly random and should not be relied upon for tasks that require high security standards. Instead use the Web Crypto API, and more precisely the window.crypto.getRandomValues() method.
Syntax
Following is the syntax of JavaScript Math.random() method −
Math.random()
Parameters
This method does not accept any parameters.
Return value
This method returns a floating-point number, pseudo-random number between 0 (inclusive) and 1 (exclusive).
Example 1
In the following example, the JavaScript Math.random() method generates a random number between 0 to 1 −
<html> <body> <script> const result = Math.random(); document.write(result); </script> </body> </html>
Output
If we execute the above program, it gives a random number between 0 to 1.
Example 2
This example generates a random decimal number between 0 (inclusive) and 10 (exclusive) −
<html> <body> <script> const result = Math.random() * 10; document.write(result); </script> </body> </html>
Output
If we execute the above program, it gives a random number between 0 to 10.
Example 3
The following example generates a random integer between 0 and 99 (inclusive) by multiplying the random decimal by 100 and rounding down using Math.floor() −
<html> <body> <script> const result = Math.floor(Math.random() * 100); document.write(result); </script> </body> </html>
Output
As we can see in the output, it generates random numbers from 0 to 99.