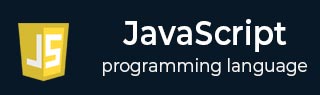
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Roadmap
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Safe Assignment Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Asynchronous
- JavaScript - Asynchronous
- JavaScript - Callback Functions
- JavaScript - Promises
- JavaScript - Async/Await
- JavaScript - Microtasks
- JavaScript - Promisification
- JavaScript - Promises Chaining
- JavaScript - Timing Events
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods & Properties
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Attributes (Attr)
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript Advanced Chapters
- JavaScript - Bubble Sort Algorithm
- JavaScript - Circular Reference Error
- JavaScript - Code Testing with Jest
- JavaScript - CORS Handling
- JavaScript - Data Analysis
- JavaScript - Dead Zone
- JavaScript - Design Patterns
- JavaScript - Engine and Runtime
- JavaScript - Execution Context
- JavaScript - Function Composition
- JavaScript - Immutability
- JavaScript - Kaboom.js
- JavaScript - Lexical Scope
- JavaScript - Local Storage
- JavaScript - Memoization
- JavaScript - Minifying JS
- JavaScript - Mutability vs Immutability
- JavaScript - Package Manager
- JavaScript - Parse S-Expressions
- JavaScript - Prototypal Inheritance
- JavaScript - Reactivity
- JavaScript - Require Function
- JavaScript - Selection API
- JavaScript - Session Storage
- JavaScript - SQL CRUD Operations
- JavaScript - Supercharged Sorts
- JavaScript - Temporal Dead Zone
- JavaScript - Throttling
- JavaScript - TRPC Library
- JavaScript - Truthy and Falsy Values
- JavaScript - Upload Files
- JavaScript - Date Comparison
- JavaScript - Recursion
- JavaScript - Data Structures
- JavaScript - Base64 Encoding
- JavaScript - Callback Function
- JavaScript - Current Date/Time
- JavaScript - Date Validation
- JavaScript - Filter Method
- JavaScript - Generating Colors
- JavaScript - HTTP Requests
- JavaScript - Insertion Sort
- JavaScript - Lazy Loading
- JavaScript - Linked List
- JavaScript - Nested Loop
- JavaScript - Null Checking
- JavaScript - Get Current URL
- JavaScript - Graph Algorithms
- JavaScript - Higher Order Functions
- JavaScript - Empty String Check
- JavaScript - Form Handling
- JavaScript - Functional Programming
- JavaScript - Parameters vs Arguments
- JavaScript - Prototype
- JavaScript - Reactive Programming
- JavaScript - Reduce Method
- JavaScript - Rest Operator
- JavaScript - Short Circuiting
- JavaScript - Undefined Check
- JavaScript - Unit Testing
- JavaScript - Validate URL
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
JavaScript Math.exp() Method
The Math.exp() method in JavaScript is used to return the result of raising Euler's number (approximately equal to 2.718) to the power of a specified number. It calculates the exponential value of the provided number, where Euler's number raised to the power of x is denoted as e^x.
The formula for calculating Euler's number raised to the power of x −
Math.exp(x) = e^x
where "e" is Euler's number and "x" is the number for which the exponential value is to be calculated.
Syntax
Following is the syntax of JavaScript Math.exp() method −
Math.exp(x)
Parameters
This method accepts only one parameter. The same is described below −
- x: A numeric value.
Return value
This method returns the value of e raised to the power of x.
Example 1
In the following example, we are using the JavaScript Math.exp() method to calculate "e" raised to the power of 2.
<html> <body> <script> const result = Math.exp(2); document.write(result); </script> </body> </html>
Output
If we execute the above program, it returns "7.3890" as result.
Example 2
In this example, we are computing "e" raised to the power of -1 −
<html> <body> <script> const result = Math.exp(-1); document.write(result); </script> </body> </html>
Output
If we execute the above program, it will return 0.3678 as result.
Example 3
In this example, we are calculating e raised to the power of 0 −
<html> <body> <script> const result = Math.exp(0); document.write(result); </script> </body> </html>
Output
The result will be 1, as any number raised to the power of 0 is 1.
Example 4
Here, we are passing "Infinity" and "-Infinity" as arguments to this method −
<html> <body> <script> const result1 = Math.exp(Infinity); const result2 = Math.exp(-Infinity); document.write(result1, <br>, result2); </script> </body> </html>
Output
If we execute the above program, it returns Infinity and 0 as result, respectively.