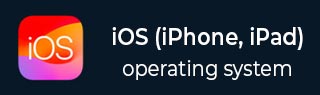
- iOS Tutorial
- iOS - Home
- iOS - Getting Started
- iOS - Environment Setup
- iOS - Objective-C Basics
- iOS - First iPhone Application
- iOS - Actions and Outlets
- iOS - Delegates
- iOS - UI Elements
- iOS - Accelerometer
- iOS - Universal Applications
- iOS - Camera Management
- iOS - Location Handling
- iOS - SQLite Database
- iOS - Sending Email
- iOS - Audio & Video
- iOS - File Handling
- iOS - Accessing Maps
- iOS - In-App Purchase
- iOS - iAd Integration
- iOS - GameKit
- iOS - Storyboards
- iOS - Auto Layouts
- iOS - Twitter & Facebook
- iOS - Memory Management
- iOS - Application Debugging
- iOS Useful Resources
- iOS - Quick Guide
- iOS - Useful Resources
- iOS - Discussion
iOS - Navigation Bar
Use of Navigation Bar
Navigation bar contains the navigation buttons of a navigation controller, which is a stack of view controllers which can be pushed and popped. Title on the navigation bar is the title of the current view controller.
Sample Code and Steps
Step 1 − Create a view based application.
Step 2 − Now, select the App Delegate.h and add a property for navigation controller as follows −
#import <UIKit/UIKit.h> @class ViewController; @interface AppDelegate : UIResponder <UIApplicationDelegate> @property (strong, nonatomic) UIWindow *window; @property (strong, nonatomic) ViewController *viewController; @property (strong, nonatomic) UINavigationController *navController; @end
Step 3 − Now update the application:didFinishLaunchingWithOptions: method in AppDelegate.m file, to allocate the navigation controller and makes it window's root view controller as follows −
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { self.window = [[UIWindow alloc] initWithFrame: [[UIScreen mainScreen] bounds]]; // Override point for customization after application launch. self.viewController = [[ViewController alloc] initWithNibName:@"ViewController" bundle:nil]; //Navigation controller init with ViewController as root UINavigationController *navController = [[UINavigationController alloc] initWithRootViewController:self.viewController]; self.window.rootViewController = navController; [self.window makeKeyAndVisible]; return YES; }
Step 4 − Add a new class file TempViewController by selecting File → New →File... → Objective C Class and then name the Class as TempViewController with subclass UIViewController.
Step 5 − Add a UIButton navButon in ViewController.h as follows −
// ViewController.h #import <UIKit/UIKit.h> @interface ViewController : UIViewController { UIButton *navButton; } @end
Step 6 − Add a method addNavigationBarItem and call the method in viewDidLoad.
Step 7 − Create a method for navigation item action.
Step 8 − We also need to create another method to push another view controller TempViewController.
Step 9 − The updated ViewController.m is as follows −
// ViewController.m #import "ViewController.h" #import "TempViewController.h" @interface ViewController () @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; [self addNavigationBarButton]; //Do any additional setup after loading the view, typically from a nib } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. } -(IBAction)pushNewView:(id)sender { TempViewController *tempVC =[[TempViewController alloc] initWithNibName:@"TempViewController" bundle:nil]; [self.navigationController pushViewController:tempVC animated:YES]; } -(IBAction)myButtonClicked:(id)sender { // toggle hidden state for navButton [navButton setHidden:!nav.hidden]; } -(void)addNavigationBarButton { UIBarButtonItem *myNavBtn = [[UIBarButtonItem alloc] initWithTitle: @"MyButton" style:UIBarButtonItemStyleBordered target: self action:@selector(myButtonClicked:)]; [self.navigationController.navigationBar setBarStyle:UIBarStyleBlack]; [self.navigationItem setRightBarButtonItem:myNavBtn]; // create a navigation push button that is initially hidden navButton = [UIButton buttonWithType:UIButtonTypeRoundedRect]; [navButton setFrame:CGRectMake(60, 50, 200, 40)]; [navButton setTitle:@"Push Navigation" forState:UIControlStateNormal]; [navButton addTarget:self action:@selector(pushNewView:) forControlEvents:UIControlEventTouchUpInside]; [self.view addSubview:navButton]; [navButton setHidden:YES]; } @end
Step 10 − When we run the application we'll get the following output −

Step 11 − On clicking the navigation button MyButton, the push navigation button visibility is toggled.
Step 12 − On clicking the push navigation button, another view controller is pushed as shown below.

To Continue Learning Please Login