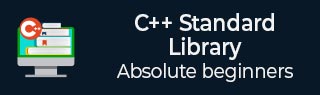
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Unordered_set::erase() function
The C++ std::unordered_set::erase() function is used to remove the specified element from the unordered_set container, either a single element or a range of elements.
This function has 3 polymorphic variants: with using the erase by position/iterator, erase by range and erase by key (you can find the syntaxes of all the variants below).
Syntax
Following is the syntax of std::unordered_set::erase() function.
iterator erase ( const_iterator position ); or size_type erase ( const key_type& k ); or iterator erase ( const_iterator first, const_iterator last );
Parameters
- position − It indicates iterator pointing to a single element to be removed.
- k − It indicates the value of element to be removed.
- (first, last) − It specify a range within the unordered_set.
Return Value
The function returns the member type iterator is a forward iterator type.
Example 1
Consider the following example, where we are going to demonstrate the usage of unordered_set::erase() function.
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<string> uSet = {"USA","Canada","France","UK","Japan","Germany","Italy"}; uSet.erase ( uSet.begin() ); uSet.erase ( "France" ); uSet.erase ( uSet.find("Japan"), uSet.end() ); cout << "uSet contains:"; for ( const string& x: uSet ) cout << " " << x; cout << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
uSet contains: Germany
Example 2
Let's look at the following example, where we are going to use the erase()function to remove the odd element from the container.
#include <unordered_set> #include <iostream> using namespace std; int main() { unordered_set<int> uSet = {10, 11, 12, 15, 17, 18}; cout << "After erasing all odd numbers: "; for (auto it = uSet.begin(); it != uSet.end();) { if (*it % 2 != 0) it = uSet.erase(it); else ++it; } cout<<"{ "; for(auto elem: uSet){ cout<<elem<<" "; } cout<<"}"; return 0; }
Output
If we run the above code it will generate the following output −
After erasing all odd numbers: { 18 12 10 }
Example 3
In the following example, we are going to erase the specified elements by using the erase() function.
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<int> uSet = {10, 20, 30, 40, 50}; uSet.erase ( 30 ); uSet.erase ( 40 ); cout << "uSet contains:"; for ( auto it: uSet ) cout << " " << it; cout << endl; return 0; }
Output
Following is the output of the above code −
uSet contains: 50 20 10
Example 4
Following is the example, where we are going to erase the elements in the range.
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<char> uSet = {'a', 'b', 'c', 'd', 'e'}; cout <<"uSet contains:"; for ( auto it: uSet ) cout <<" " <<it; cout <<endl; uSet.erase ( uSet.begin(), uSet.find('c') ); cout <<"after erased uSet contains:"; for ( auto it: uSet ) cout <<" " <<it; cout <<endl; return 0; }
Ouput
Output of the above code is as follows −
uSet contains: e d c b a after erased uSet contains: c b a
To Continue Learning Please Login