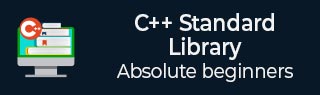
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Unordered_set:: begin() Function
The C++ std::unordered_set::begin() function is used to return an iterator pointing to the first element of the unordered_set container. If the unordered_set is empty, the returned iterator will be equal to the end()
An iterator is an object (like a pointer) over elements that provides access to each individual element. Iterators can be used to modify pointed elements available in the unordered_set container.
Syntax
Following is the syntax of std::unordered_set::begin() function.
iterator begin() noexcept; const_iterator begin() const noexcept; or local_iterator begin ( size_type n ); const_local_iterator begin ( size_type n ) const;
Parameters
- n &minus It indicates the bucket number that must be less than bucket_count.
Return value
This function returns an iterator pointing to the first element in the unordered_set container or an iterator pointing to the first element of the specified bucket.
Example 1
Let's look at the following example, where we are going to demonstrate the usage of unordered_set::begin() function.
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<string> myUset = {"100","200","300","400","500","600","700","800"}; cout<<"Contents of the myUset are: "<<endl; for(auto it: myUset) cout<<it<<" "; cout<<"\nAn iterator of the first is: "; auto it = myUset.begin(); cout<<*it; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Contents of the myUset are: 700 600 500 800 400 300 200 100 An iterator of the first is: 700
Example 2
Consider the following example, where we are going to use the begin() function inside a for loop to display the elements of the container.
#include <iostream> #include <string> #include <unordered_set> int main () { std::unordered_set<std::string> myUset = {"100","200","300","400","500"}; std::cout << "myUset contains:"; for ( auto it = myUset.begin(); it != myUset.end(); ++it ) std::cout << " " << *it; std::cout << std::endl; return 0; }
Output
If we run the above code it will generate the following output −
myUset contains: 500 400 300 200 100
Example 3
In the following example, we are going to use the begin() function that accepts i as a parameter to return the element of each bucket.
#include <iostream> #include <string> #include <unordered_set> int main () { std::unordered_set<std::string> myUset = {"100", "200", "300", "400", "500"}; std::cout << "myUset's buckets contain:\n"; for ( unsigned i = 0; i < myUset.bucket_count(); ++i) { std::cout << "bucket #" << i << " contains:"; for ( auto local_it = myUset.begin(i); local_it!= myUset.end(i); ++local_it ) std::cout << " " << *local_it; std::cout << std::endl; } return 0; }
Output
Following is the output of the above code −
myUset's buckets contain: bucket #0 contains: bucket #1 contains: 400 bucket #2 contains: 500 bucket #3 contains: bucket #4 contains: 100 bucket #5 contains: bucket #6 contains: bucket #7 contains: bucket #8 contains: bucket #9 contains: bucket #10 contains: 300 bucket #11 contains: 200 bucket #12 contains:
Example 4
Following is the another example of the usage of begin() function to get the iterator pointing to the first element of the specified bucket.
#include <iostream> #include <string> #include <unordered_set> using namespace std; int main () { unordered_set<int> myUset = {10, 20, 30, 40, 50}; cout << "Iterator pointing to the first element of the bucket 4 is: "; auto it = myUset.begin(4); cout<<*it<<endl; return 0; }
Output
Output of the above code is as follows −
Iterator pointing to the first element of the bucket 4 is: 30
To Continue Learning Please Login