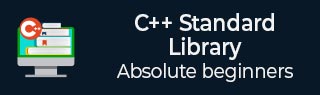
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ unordered_multimap::swap() Function
The C++ std::unordered_multimap::swap() function is used to exchange the content of the first unordered_multimap with another. It will take place when the other container is of the same type.
This function exchanges the element or key/value pairs without actually performing any copies or moves on the individual element, allowing for constant-time execution no matter the size.
Syntax
Following is the syntax of std::unordered_multimap::swap() function.
void swap(unordered_multimap& n);
Parameters
- n − It indicates the another unordered_multimap object.
Return value
This function does not returns anything.
Example 1
Let's look at the following example, where we are going to demonstrate the usage of unordered_multimap::swap() function.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm1 = { {'a', 1}, {'a', 10}, {'a', 100}, {'a', 1000}, {'a', 10000} }; unordered_multimap<char, int> umm2; umm1.swap(umm2); cout << "second unordered_multimap contains following elements after swap " << endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
Output
If we run the above code it will generate the following output −
second unordered_multimap contains following elements after swap a = 10000 a = 1000 a = 100 a = 10 a = 1
Example 2
Consider the following example, where we are going to use the swap() function and swap the elements from one container to another container, vice-versa.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm1 = { {'a', 1}, {'b', 2}, {'c', 3}, {'b', 20}, {'c', 30}, }; unordered_multimap<char, int> umm2 = { {'e', 5}, {'f', 6}, {'g', 7}, {'h', 8} }; cout<<"umm1 contains following element before swap: "<<endl; for(auto & it:umm1){ cout<<it.first<< " = "<<it.second<<endl; } cout<<"umm2 contains following element before swap: "<<endl; for(auto & it:umm2){ cout<<it.first<< " = "<<it.second<<endl; } umm1.swap(umm2); cout << "umm1 contains following elements after swap: " << endl; for (auto it = umm1.begin(); it != umm1.end(); ++it) cout << it->first << " = " << it->second << endl; cout << "umm2 contains following elements after swap: " << endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
Output
Following is the output of the above code −
umm1 contains following element before swap: c = 30 c = 3 b = 20 b = 2 a = 1 umm2 contains following element before swap: h = 8 g = 7 f = 6 e = 5 umm1 contains following elements after swap: h = 8 g = 7 f = 6 e = 5 umm2 contains following elements after swap: c = 30 c = 3 b = 20 b = 2 a = 1
Example 3
In the following example, we are going to use the swap() function to swap only the only even key and its value from one unordered_multimap to another unordered_multimap.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<int, int> umm1, umm2, temp; umm1 = {{1, 10}, {2, 20}, {1, 100}, {2, 200}, {3, 300}, {4, 40}}; cout<<"umm1 contains following element before swap: "<<endl; for(auto& it:umm1){ cout<<it.first<<" = "<<it.second<<endl; } for(auto & it:umm1){ if(it.first%2 == 0){ temp.insert({it.first, it.second}); } } umm2.swap(temp); cout<<"umm2 contains following element before swap: "<<endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
Output
Output of the above code is as follows −
umm1 contains following element before swap: 4 = 40 3 = 300 2 = 200 2 = 20 1 = 100 1 = 10 umm2 contains following element before swap: 2 = 20 2 = 200 4 = 40
To Continue Learning Please Login