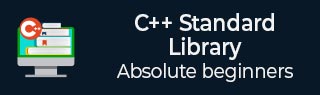
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ unordered_multimap::max_bucket_count() Function
The C++ std::unordered_multimap::max_bucket_count() function is used to return the maximum number of buckets that the unordered_multimap container can hold, based on its current memory allocation. each bucket in an unordered_multimap is a container that holds element with same hash value.
A bucket is a slot in the container's internal hash table to which elements are assigned based on the hash value of their key.
Syntax
Following is the syntax of the std::unordered_multimap:: max_bucket_count() function.
size_type max_bucket_count() const;
Parameters
This function does not accepts any parameter.
Return value
This function returns an unsigned integer that represent the maximum number of buckets.
Example 1
Let's look at the following example, where we are going to use the max_bucket_count() function on empty multimap and observing the output.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> um; cout << "max_bucket_count of unordered_multimap = " << um.max_bucket_count() << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
max_bucket_count of unordered_multimap = 576460752303423487
Example 2
Consider the another scenario, where we are going to get the maximum number of buckets in the current multimap using the max_bucket_count.
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm={{'A', 1}, {'B', 2}, {'A', 3}, {'B', 4}, {'C', 5}}; cout << "max_bucket_count of unordered_multimap = " << umm.max_bucket_count() << endl; return 0; }
Output
If we run the above code it will generate the following output −
max_bucket_count of unordered_multimap = 576460752303423487
Example 3
In the following example, we are creating an unordered_multimap that stores the negative key value and then using the max_bucket_count to get the maximum number of bucket, as follows −
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<int, char> umm={{-1, 'a'}, {-2, 'b'}, {-3, 'c'}, {-2, 'd'}, {-3, 'e'}}; cout << "max_bucket_count of unordered_multimap = " << umm.max_bucket_count() << endl; return 0; }
Output
Following is the output of the above code −
max_bucket_count of unordered_multimap = 576460752303423487
Example 4
In the following example, we are going to get the maximum bucket count before and after the insertion of elements into the empty multimap.
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_multimap<char, int> ummap; cout << "Size is : " << ummap.size() << endl; cout << "Max bucket count is : " << ummap.max_bucket_count() << endl; // insert elements ummap.insert({ 'a', 10 }); ummap.insert({ 'b', 12 }); ummap.insert({ 'c', 13 }); ummap.insert({ 'b', 12 }); ummap.insert({ 'd', 15 }); ummap.insert({ 'e', 20 }); cout << "Size is : " << ummap.size() << endl; cout << "Max bucket count is : " << ummap.max_bucket_count() << endl; return 0; }
Output
Output of the above code is as follows −
Size is : 0 Max bucket count is : 576460752303423487 Size is : 6 Max bucket count is : 576460752303423487
To Continue Learning Please Login