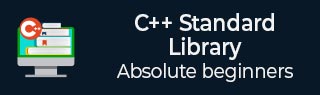
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ List::reverse() Function
The C++ std::list::reverse() function is used to reverse the order of the elements in the list.
It does not accept any parameter and reverses the order of the elements in the list container. For example, suppose we have a list with the value {1,2,3,4}, if we try to reverse the order of the list elements, the last element is placed in the first position, the second last element is placed in the second position, and so on. Finally, the output will be displayed as {4,3,2,1}. The return type of this function is void which implies that it does not return any value.
Syntax
Following is the syntax of the C++ std::list::void reverse() function −
void reverse();
Parameters
- It does not accept any parameter.
Return Value
This function does not return any value.
Example 1
In the following program, we are using the C++ std::list::reverse() function to reverse the order of the elements in the current list {10,20, 30, 40}.
#include<iostream> #include<list> using namespace std; int main() { list<int> num_list = {10,20, 30, 40}; cout<<"The list elements before the reverse() operation: "<<endl; for(int lst : num_list) { cout<<lst<<" "; } //use the reverse() function num_list.reverse(); cout<<"\nThe list elements after the reverse() operation: "; for(int lst1 : num_list) { cout<<lst1<<" "; } }
Output
The above program produces the following output −
The list elements before the reverse() operation: 10 20 30 40 The list elements after the reverse() operation: 40 30 20 10
Example 2
Apart from the int elements, you can also reverse the order of char elements in the list(type char).
Following is another example of the C++ std::list::reverse() function. Here, we are creating a list(type char) with the elements {'O', 'L', 'L', 'H', 'E'}. Then, using the reverse() function, we are trying to reverse the order of the elements in the current list.
#include<iostream> #include<list> using namespace std; int main() { list<char> char_list = {'O', 'L', 'L', 'E', 'H'}; cout<<"The list elements before the reverse() operation: "<<endl; for(char lst : char_list) { cout<<lst<<" "; } //using the reverse() function char_list.reverse(); cout<<"\nThe list elements after the reverse() operation: "; for(char lst1 : char_list) { cout<<lst1<<" "; } }
Output
On executing the above program, it will produce the following output −
The list elements before the reverse() operation: O L L E H The list elements after the reverse() operation: H E L L O
Example 3
You can also reverse the order of string elements in the list(type string).
In this program, we create a list{type string) with the elements {"Welcome", "to", "Tutorials", "Point"}. Then, using the reverse() function, we are trying to reverse the order of elements in this list.
#include<iostream> #include<list> using namespace std; int main() { list<string> msg = {"Welcome", "to", "Tutorials", "Point"}; cout<<"The list Elements before the reverse() operation: "<<endl; for(string l1: msg) { cout<<l1<<" "; } //using the reverse() function msg.reverse(); cout<<"\nThe list elements after the reverse() function: "; for(string l2: msg){ cout<<l2<<" "; } return 0; }
Output
This will generate the following output −
The list Elements before the reverse() operation: Welcome to Tutorials Point The list elements after the reverse() function: Point Tutorials to Welcome
To Continue Learning Please Login