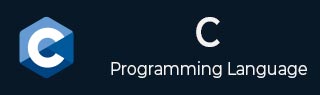
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <float.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdarg.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <time.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library function - fwrite()
Description
The C library function size_t fwrite(const void *ptr, size_t size, size_t nmemb, FILE *stream) writes data from the array pointed to, by ptr to the given stream.
Declaration
Following is the declaration for fwrite() function.
size_t fwrite(const void *ptr, size_t size, size_t nmemb, FILE *stream)
Parameters
ptr − This is the pointer to the array of elements to be written.
size − This is the size in bytes of each element to be written.
nmemb − This is the number of elements, each one with a size of size bytes.
stream − This is the pointer to a FILE object that specifies an output stream.
Return Value
This function returns the total number of elements successfully returned as a size_t object, which is an integral data type. If this number differs from the nmemb parameter, it will show an error.
Example
The following example shows the usage of fwrite() function.
#include<stdio.h> int main () { FILE *fp; char str[] = "This is tutorialspoint.com"; fp = fopen( "file.txt" , "w" ); fwrite(str , 1 , sizeof(str) , fp ); fclose(fp); return(0); }
Let us compile and run the above program that will create a file file.txt which will have following content −
This is tutorialspoint.com
Now let's see the content of the above file using the following program −
#include <stdio.h> int main () { FILE *fp; int c; fp = fopen("file.txt","r"); while(1) { c = fgetc(fp); if( feof(fp) ) { break ; } printf("%c", c); } fclose(fp); return(0); }
Let us compile and run the above program to produce the following result −
This is tutorialspoint.com
To Continue Learning Please Login