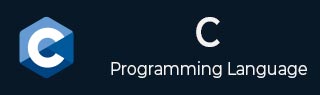
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C Library - isalnum() function
The C ctype library isalnum() function is used to check if a given character is alphanumeric, which means it is either a letter (uppercase or lowercase) or a digit.
In order to use isalnum() function we have to include the header file <ctype.h> that contains the function.
Syntax
Following is the C library syntax of the isalnum() function −
int isalnum(int c);
Parameters
This function accepts a single parameter −
c − This parameter represents the character to be checked. It is passed as an int, but it is expected to be a character that can be represented as an unsigned char or the value of EOF
Return Value
Following is the return value −
The function returns a non-zero value (true) if the character is alphanumeric.
It returns 0 (false) if the character is not alphanumeric.
Example 1: Checking if a Character is Alphanumeric
In this example, the character a is checked using the isalnum() function. Since a is an alphabetic letter, the function returns a non-zero value, indicating it is alphanumeric.
#include <stdio.h> #include <ctype.h> int main() { char ch = 'a'; if (isalnum(ch)) { printf("The character '%c' is alphanumeric.\n", ch); } else { printf("The character '%c' is not alphanumeric.\n", ch); } return 0; }
Output
The above code produces following result −
The character 'a' is alphanumeric.
Example 2: Checking a Special Character
Now, the special character @ is checked. Since it is neither a letter nor a digit, the isalnum function returns 0, indicating that @ is not alphanumeric.
#include <stdio.h> #include <ctype.h> int main() { char ch = '@'; if (isalnum(ch)) { printf("The character '%c' is alphanumeric.\n", ch); } else { printf("The character '%c' is not alphanumeric.\n", ch); } return 0; }
Output
The output of the above code is as follows −
The character '@' is not alphanumeric.