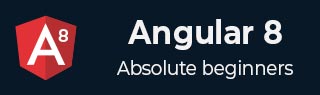
- Angular 8 Tutorial
- Angular 8 - Home
- Angular 8 - Introduction
- Angular 8 - Installation
- Creating First Application
- Angular 8 - Architecture
- Angular Components and Templates
- Angular 8 - Data Binding
- Angular 8 - Directives
- Angular 8 - Pipes
- Angular 8 - Reactive Programming
- Services and Dependency Injection
- Angular 8 - Http Client Programming
- Angular 8 - Angular Material
- Routing and Navigation
- Angular 8 - Animations
- Angular 8 - Forms
- Angular 8 - Form Validation
- Authentication and Authorization
- Angular 8 - Web Workers
- Service Workers and PWA
- Angular 8 - Server Side Rendering
- Angular 8 - Internationalization (i18n)
- Angular 8 - Accessibility
- Angular 8 - CLI Commands
- Angular 8 - Testing
- Angular 8 - Ivy Compiler
- Angular 8 - Building with Bazel
- Angular 8 - Backward Compatibility
- Angular 8 - Working Example
- Angular 9 - What’s New?
- Angular 8 Useful Resources
- Angular 8 - Quick Guide
- Angular 8 - Useful Resources
- Angular 8 - Discussion
Angular 8 - Pipes
Pipes are referred as filters. It helps to transform data and manage data within interpolation, denoted by {{ | }}. It accepts data, arrays, integers and strings as inputs which are separated by ‘|’ symbol. This chapter explains about pipes in detail.
Adding parameters
Create a date method in your test.component.ts file.
export class TestComponent { presentDate = new Date(); }
Now, add the below code in your test.component.html file.
<div> Today's date :- {{presentDate}} </div>
Now, run the application, it will show the following output −
Today's date :- Mon Jun 15 2020 10:25:05 GMT+0530 (IST)
Here,
Date object is converted into easily readable format.
Add Date pipe
Let’s add date pipe in the above html file.
<div> Today's date :- {{presentDate | date }} </div>
You could see the below output −
Today's date :- Jun 15, 2020
Parameters in Date
We can add parameter in pipe using : character. We can show short, full or formatted dates using this parameter. Add the below code in test.component.html file.
<div> short date :- {{presentDate | date:'shortDate' }} <br/> Full date :- {{presentDate | date:'fullDate' }} <br/> Formatted date:- {{presentDate | date:'M/dd/yyyy'}} <br/> Hours and minutes:- {{presentDate | date:'h:mm'}} </div>
You could see the below response on your screen −
short date :- 6/15/20 Full date :- Monday, June 15, 2020 Formatted date:- 6/15/2020 Hours and minutes:- 12:00
Chained pipes
We can combine multiple pipes together. This will be useful when a scenario associates with more than one pipe that has to be applied for data transformation.
In the above example, if you want to show the date with uppercase letters, then we can apply both Date and Uppercase pipes together.
<div> Date with uppercase :- {{presentDate | date:'fullDate' | uppercase}} <br/> Date with lowercase :- {{presentDate | date:'medium' | lowercase}} <br/> </div>
You could see the below response on your screen −
Date with uppercase :- MONDAY, JUNE 15, 2020 Date with lowercase :- jun 15, 2020, 12:00:00 am
Here,
Date, Uppercase and Lowercase are pre-defined pipes. Let’s understand other types of built-in pipes in next section.
Built-in Pipes
Angular 8 supports the following built-in pipes. We will discuss one by one in brief.
AsyncPipe
If data comes in the form of observables, then Async pipe subscribes to an observable and returns the transmitted values.
import { Observable, Observer } from 'rxjs'; export class TestComponent implements OnInit { timeChange = new Observable<string>((observer: Observer>string>) => { setInterval(() => observer.next(new Date().toString()), 1000); }); }
Here,
The Async pipe performs subscription for time changing in every one seconds and returns the result whenever gets passed to it. Main advantage is that, we don’t need to call subscribe on our timeChange and don’t worry about unsubscribe, if the component is removed.
Add the below code inside your test.component.html.
<div> Seconds changing in Time: {{ timeChange | async }} </div>
Now, run the application, you could see the seconds changing on your screen.
CurrencyPipe
It is used to convert the given number into various countries currency format. Consider the below code in test.component.ts file.
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-test', template: ` <div style="text-align:center"> <h3> Currency Pipe</h3> <p>{{ price | currency:'EUR':true}}</p> <p>{{ price | currency:'INR' }}</p> </div> `, styleUrls: ['./test.component.scss'] }) export class TestComponent implements OnInit { price : number = 20000; ngOnInit() { } }
You could see the following output on your screen −
Currency Pipe €20,000.00 ₹20,000.00
SlicePipe
Slice pipe is used to return a slice of an array. It takes index as an argument. If you assign only start index, means it will print till the end of values. If you want to print specific range of values, then we can assign start and end index.
We can also use negative index to access elements. Simple example is shown below −
test.component.ts
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-test', template: ` <div> <h3>Start index:- {{Fruits | slice:2}}</h3> <h4>Start and end index:- {{Fruits | slice:1:4}}</h4> <h5>Negative index:- {{Fruits | slice:-2}}</h5> <h6>Negative start and end index:- {{Fruits | slice:-4:-2}}</h6> </div> `, styleUrls: ['./test.component.scss'] }) export class TestComponent implements OnInit { Fruits = ["Apple","Orange","Grapes","Mango","Kiwi","Pomegranate"]; ngOnInit() { } }
Now run your application and you could see the below output on your screen −
Start index:- Grapes,Mango,Kiwi,Pomegranate Start and end index:- Orange,Grapes,Mango Negative index:- Kiwi,Pomegranate Negative start and end index:- Grapes,Mango
Here,
{{Fruits | slice:2}} means it starts from second index value Grapes to till the end of value.
{{Fruits | slice:1:4}} means starts from 1 to end-1 so the result is one to third index values.
{{Fruits | slice:-2}} means starts from -2 to till end because no end value is specified. Hence the result is Kiwi, Pomegranate.
{{Fruits | slice:-4:-2}} means starts from negative index -4 is Grapes to end-1 which is -3 so the result of index[-4,-3] is Grapes, Mango.
DecimalPipe
It is used to format decimal values. It is also considered as CommonModule. Let’s understand a simple code in test.component.ts file,
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-test', template: ` <div style="text-align:center"> <h3>Decimal Pipe</h3> <p> {{decimalNum1 | number}} </p> <p> {{decimalNum2 | number}} </p> </div> `, styleUrls: ['./test.component.scss'] }) export class TestComponent implements OnInit { decimalNum1: number = 8.7589623; decimalNum2: number = 5.43; ngOnInit() { } }
You could see the below output on your screen −
Decimal Pipe 8.759 5.43
Formatting values
We can apply string format inside number pattern. It is based on the below format −
number:"{minimumIntegerDigits}.{minimumFractionDigits} - {maximumFractionDigits}"
Let’s apply the above format in our code,
@Component({ template: ` <div style="text-align:center"> <p> Apply formatting:- {{decimalNum1 | number:'3.1'}} </p> <p> Apply formatting:- {{decimalNum1 | number:'2.1-4'}} </p> </div> `, })
Here,
{{decimalNum1 | number:’3.1’}} means three decimal place and minimum of one fraction but no constraint about maximum fraction limit. It returns the following output −
Apply formatting:- 008.759
{{decimalNum1 | number:’2.1-4’}} means two decimal places and minimum one and maximum of four fractions allowed so it returns the below output −
Apply formatting:- 08.759
PercentPipe
It is used to format number as percent. Formatting strings are same as DecimalPipe concept. Simple example is shown below −
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-test', template: ` <div style="text-align:center"> <h3>Decimal Pipe</h3> <p> {{decimalNum1 | percent:'2.2'}} </p> </div> `, styleUrls: ['./test.component.scss'] }) export class TestComponent { decimalNum1: number = 0.8178; }
You could see the below output on your screen −
Decimal Pipe 81.78%
JsonPipe
It is used to transform a JavaScript object into a JSON string. Add the below code in test.component.ts file as follows −
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-test', template: ` <div style="text-align:center"> <p ngNonBindable>{{ jsonData }}</p> (1) <p>{{ jsonData }}</p> <p ngNonBindable>{{ jsonData | json }}</p> <p>{{ jsonData | json }}</p> </div> `, styleUrls: ['./test.component.scss'] }) export class TestComponent { jsonData = { id: 'one', name: { username: 'user1' }} }
Now, run the application, you could see the below output on your screen −
{{ jsonData }} (1) [object Object] {{ jsonData | json }} { "id": "one", "name": { "username": "user1" } }
Creating custom pipe
As we have seen already, there is a number of pre-defined Pipes available in Angular 8 but sometimes, we may want to transform values in custom formats. This section explains about creating custom Pipes.
Create a custom Pipe using the below command −
ng g pipe digitcount
After executing the above command, you could see the response −
CREATE src/app/digitcount.pipe.spec.ts (203 bytes) CREATE src/app/digitcount.pipe.ts (213 bytes) UPDATE src/app/app.module.ts (744 bytes)
Let’s create a logic for counting digits in a number using Pipe. Open digitcount.pipe.ts file and add the below code −
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'digitcount' }) export class DigitcountPipe implements PipeTransform { transform(val : number) : number { return val.toString().length; } }
Now, we have added logic for count number of digits in a number. Let’s add the final code in test.component.ts file as follows −
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-test', template: ` <div> <p> DigitCount Pipe </p> <h1>{{ digits | digitcount }}</h1> </div> `, styleUrls: ['./test.component.scss'] }) export class TestComponent implements OnInit { digits : number = 100; ngOnInit() { } }
Now, run the application, you could see the below response −
DigitCount Pipe 3
Working example
Let us use the pipe in the our ExpenseManager application.
Open command prompt and go to project root folder.
cd /go/to/expense-manager
Start the application.
ng serve
Open ExpenseEntryListComponent’s template, src/app/expense-entry-list/expense-entry-list.component.html and include pipe in entry.spendOn as mentioned below −
<td>{{ entry.spendOn | date: 'short' }}</td>
Here, we have used the date pipe to show the spend on date in the short format.
Finally, the output of the application is as shown below −

To Continue Learning Please Login